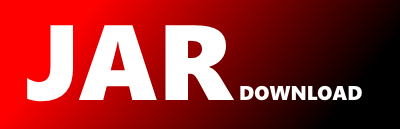
com.fasterxml.jackson.jr.ob.JSONComposer Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jackson-jr-objects Show documentation
Show all versions of jackson-jr-objects Show documentation
Simple data-binding that builds directly on jackson-core (streaming),
has no other dependencies, and provides additional builder-style content generator
package com.fasterxml.jackson.jr.ob;
import java.io.IOException;
import java.util.*;
import com.fasterxml.jackson.core.JsonGenerator;
import com.fasterxml.jackson.core.io.SegmentedStringWriter;
import com.fasterxml.jackson.core.util.ByteArrayBuilder;
import com.fasterxml.jackson.jr.ob.JSON.Feature;
import com.fasterxml.jackson.jr.ob.comp.SequenceComposer;
/**
* Root-level composer object that acts as streaming "builder"
* object, using an underlying {@link com.fasterxml.jackson.core.JsonGenerator} object.
* This is similar to {@link com.fasterxml.jackson.jr.ob.comp.ArrayComposer}, but does not
* have parent composer (so no end()
method),
* but does implement {@link java.io.Closeable}
*
* @param Type of result being composed.
*/
public class JSONComposer
extends SequenceComposer>
{
protected final int _features;
protected final boolean _closeGenerator;
protected T _result;
protected SegmentedStringWriter _stringWriter;
protected ByteArrayBuilder _byteWriter;
public JSONComposer(int features, JsonGenerator gen, boolean closeGenerator)
{
super(gen);
_features = features;
_stringWriter = null;
_byteWriter = null;
_closeGenerator = closeGenerator;
}
protected JSONComposer(int features, JsonGenerator gen, SegmentedStringWriter w)
{
super(gen);
_features = features;
_stringWriter = w;
_byteWriter = null;
_closeGenerator = true;
}
protected JSONComposer(int features, JsonGenerator gen, ByteArrayBuilder w)
{
super(gen);
_features = features;
_stringWriter = null;
_byteWriter = w;
_closeGenerator = true;
}
protected JSONComposer(int features, T result)
{
super(null);
_features = features;
_result = result;
_stringWriter = null;
_byteWriter = null;
_closeGenerator = false;
}
/*
/**********************************************************************
/* API, factory methods
/**********************************************************************
*/
public static JSONComposer streamComposer(int features,
JsonGenerator gen, boolean closeGenerator)
{
return new JSONComposer(features, gen, closeGenerator);
}
public static JSONComposer stringComposer(int features,
JsonGenerator gen, SegmentedStringWriter w) {
return new JSONComposer(features, gen, w);
}
public static JSONComposer bytesComposer(int features,
JsonGenerator gen, ByteArrayBuilder w) {
return new JSONComposer(features, gen, w);
}
public static > JSONComposer collectionComposer(int features,
T coll) {
return new JSONComposer(features, coll);
}
public static JSONComposer
© 2015 - 2024 Weber Informatics LLC | Privacy Policy