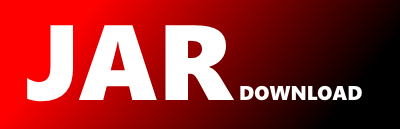
com.fasterxml.jackson.jr.ob.comp.MapComposer Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jackson-jr-objects Show documentation
Show all versions of jackson-jr-objects Show documentation
Simple data-binding that builds directly on jackson-core (streaming),
has no other dependencies, and provides additional builder-style content generator
The newest version!
package com.fasterxml.jackson.jr.ob.comp;
import java.io.IOException;
import java.util.*;
import com.fasterxml.jackson.core.SerializableString;
public class MapComposer
extends ComposerBase
{
protected final PARENT _parent;
protected String _fieldName;
protected Map _map;
public MapComposer(PARENT parent) {
super();
_parent = parent;
}
public MapComposer(Map map) {
super();
_parent = null;
_map = map;
}
@SuppressWarnings({ "unchecked", "rawtypes" })
public static MapComposer> rootComposer(Map map) {
return new MapComposer(map);
}
/*
/**********************************************************************
/* Abstract method impls
/**********************************************************************
*/
@Override
public void flush() throws IOException { }
@Override
protected MapComposer _start() {
if (_map == null) {
_map = constructMap();
}
return this;
}
@Override
protected Map _finish()
{
if (_open) {
_open = false;
}
return _map;
}
/*
/**********************************************************************
/* Compose methods, structures
/**********************************************************************
*/
public CollectionComposer,?> startArrayField(String fieldName)
{
_closeChild();
_fieldName = fieldName;
CollectionComposer,?> child = _startCollection(this);
_map.put(fieldName, child._collection);
return child;
}
public CollectionComposer,?> startArrayField(SerializableString fieldName) {
return startArrayField(fieldName.getValue());
}
public MapComposer> startObjectField(String fieldName)
{
_closeChild();
_fieldName = fieldName;
MapComposer> child = _startMap(this);
_map.put(fieldName, child._map);
return child;
}
public MapComposer> startObjectField(SerializableString fieldName) {
return startObjectField(fieldName.getValue());
}
public PARENT end()
{
_closeChild();
if (_open) {
_open = false;
_parent._childClosed();
}
return _parent;
}
public Map finish() {
return _finish();
}
/*
/**********************************************************************
/* Compose methods, scalars
/**********************************************************************
*/
public MapComposer put(String fieldName, boolean value)
{
_map.put(fieldName, value ? Boolean.TRUE : Boolean.FALSE);
return this;
}
public MapComposer putNull(String fieldName)
{
// could maybe just omit but...
_map.put(fieldName, null);
return this;
}
public MapComposer put(String fieldName, int value)
{
_map.put(fieldName, value);
return this;
}
public MapComposer put(String fieldName, long value)
{
_map.put(fieldName, value);
return this;
}
public MapComposer put(String fieldName, double value)
{
_map.put(fieldName, value);
return this;
}
public MapComposer put(String fieldName, String value)
{
_map.put(fieldName, value);
return this;
}
public MapComposer put(String fieldName, CharSequence value)
{
String str = (value == null) ? null : value.toString();
_map.put(fieldName, str);
return this;
}
/*
/**********************************************************************
/* Overridable helper methods
/**********************************************************************
*/
protected Map constructMap() {
return new LinkedHashMap();
}
/*
/**********************************************************************
/* Internal methods
/**********************************************************************
*/
protected void _closeChild()
{
if (_child != null) {
Object value = _child._safeFinish();
_map.put(_fieldName, value);
_child = null;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy