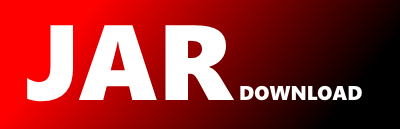
com.fasterxml.jackson.jr.ob.comp.ObjectComposer Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jackson-jr-objects Show documentation
Show all versions of jackson-jr-objects Show documentation
Simple data-binding that builds directly on jackson-core (streaming),
has no other dependencies, and provides additional builder-style content generator
The newest version!
package com.fasterxml.jackson.jr.ob.comp;
import java.io.IOException;
import com.fasterxml.jackson.core.JsonGenerator;
import com.fasterxml.jackson.core.JsonProcessingException;
import com.fasterxml.jackson.core.SerializableString;
public class ObjectComposer
extends ComposerBase
{
protected final PARENT _parent;
protected final JsonGenerator _generator;
public ObjectComposer(PARENT parent, JsonGenerator g) {
super();
_parent = parent;
_generator = g;
}
/*
/**********************************************************************
/* Abstract method impls
/**********************************************************************
*/
@Override
public void flush() throws IOException {
if (_generator != null) {
_generator.close();
}
}
@Override
protected ObjectComposer _start() throws IOException, JsonProcessingException {
_generator.writeStartObject();
return this;
}
@Override
protected Object _finish() throws IOException, JsonProcessingException
{
if (_open) {
_open = false;
_generator.writeEndObject();
}
return null;
}
/*
/**********************************************************************
/* Compose methods, structures
/**********************************************************************
*/
public ArrayComposer> startArrayField(String fieldName)
throws IOException, JsonProcessingException
{
_closeChild();
_generator.writeFieldName(fieldName);
return _startArray(this, _generator);
}
public ArrayComposer> startArrayField(SerializableString fieldName)
throws IOException, JsonProcessingException
{
_closeChild();
_generator.writeFieldName(fieldName);
return _startArray(this, _generator);
}
public ObjectComposer> startObjectField(String fieldName)
throws IOException, JsonProcessingException
{
_closeChild();
_generator.writeFieldName(fieldName);
return _startObject(this, _generator);
}
public ObjectComposer> startObjectField(SerializableString fieldName)
throws IOException, JsonProcessingException
{
_closeChild();
_generator.writeFieldName(fieldName);
return _startObject(this, _generator);
}
public PARENT end()
throws IOException, JsonProcessingException
{
_closeChild();
if (_open) {
_open = false;
_generator.writeEndObject();
_parent._childClosed();
}
return _parent;
}
/*
/**********************************************************************
/* Compose methods, scalars
/**********************************************************************
*/
public ObjectComposer put(String fieldName, boolean value)
throws IOException, JsonProcessingException
{
_generator.writeBooleanField(fieldName, value);
return this;
}
public ObjectComposer putNull(String fieldName)
throws IOException, JsonProcessingException
{
_generator.writeNullField(fieldName);
return this;
}
/**
* Method used to put a Java Object ("POJO") value into Object being
* composed: this requires that the underlying {@link JsonGenerator}
* has a properly configured {@link com.fasterxml.jackson.core.ObjectCodec}
* to use for serializer object.
*
* @since 2.6
*/
public ObjectComposer putObject(String fieldName, Object value)
throws IOException, JsonProcessingException
{
_generator.writeObjectField(fieldName, value);
return this;
}
public ObjectComposer put(String fieldName, int value)
throws IOException, JsonProcessingException
{
_generator.writeNumberField(fieldName, value);
return this;
}
public ObjectComposer put(String fieldName, long value)
throws IOException, JsonProcessingException
{
_generator.writeNumberField(fieldName, value);
return this;
}
public ObjectComposer put(String fieldName, double value)
throws IOException, JsonProcessingException
{
_generator.writeNumberField(fieldName, value);
return this;
}
public ObjectComposer put(String fieldName, String value)
throws IOException, JsonProcessingException
{
_generator.writeStringField(fieldName, value);
return this;
}
public ObjectComposer put(String fieldName, CharSequence value)
throws IOException, JsonProcessingException
{
String str = (value == null) ? null : value.toString();
_generator.writeStringField(fieldName, str);
return this;
}
/*
/**********************************************************************
/* Internal methods
/**********************************************************************
*/
protected void _closeChild()
throws IOException, JsonProcessingException
{
if (_child != null) {
_child._finish();
_child = null;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy