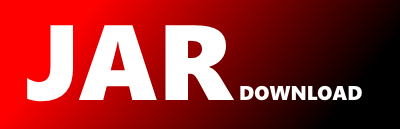
com.fasterxml.jackson.module.kotlin.Converters.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jackson-module-kotlin Show documentation
Show all versions of jackson-module-kotlin Show documentation
Add-on module for Jackson (https://github.com/FasterXML/jackson/) to support
Kotlin language, specifically introspection of method/constructor parameter names,
without having to add explicit property name annotation.
package com.fasterxml.jackson.module.kotlin
import com.fasterxml.jackson.databind.JavaType
import com.fasterxml.jackson.databind.deser.std.StdDelegatingDeserializer
import com.fasterxml.jackson.databind.ser.std.StdDelegatingSerializer
import com.fasterxml.jackson.databind.type.TypeFactory
import com.fasterxml.jackson.databind.util.StdConverter
import kotlin.reflect.KClass
import kotlin.time.toJavaDuration
import kotlin.time.toKotlinDuration
import java.time.Duration as JavaDuration
import kotlin.time.Duration as KotlinDuration
internal class SequenceToIteratorConverter(private val input: JavaType) : StdConverter, Iterator<*>>() {
override fun convert(value: Sequence<*>): Iterator<*> = value.iterator()
override fun getInputType(typeFactory: TypeFactory): JavaType = input
// element-type may not be obtained, so a null check is required
override fun getOutputType(typeFactory: TypeFactory): JavaType = input.containedType(0)
?.let { typeFactory.constructCollectionLikeType(Iterator::class.java, it) }
?: typeFactory.constructType(Iterator::class.java)
}
internal object KotlinDurationValueToJavaDurationConverter : StdConverter() {
private val boxConverter by lazy { ValueClassBoxConverter(Long::class.java, KotlinDuration::class) }
override fun convert(value: Long): JavaDuration = KotlinToJavaDurationConverter.convert(boxConverter.convert(value))
}
internal object KotlinToJavaDurationConverter : StdConverter() {
override fun convert(value: KotlinDuration) = value.toJavaDuration()
}
/**
* Currently it is not possible to deduce type of [kotlin.time.Duration] fields therefore explicit annotation is needed on fields in order to properly deserialize POJO.
*
* @see [com.fasterxml.jackson.module.kotlin.test.DurationTests]
*/
internal object JavaToKotlinDurationConverter : StdConverter() {
override fun convert(value: JavaDuration) = value.toKotlinDuration()
val delegatingDeserializer: StdDelegatingDeserializer by lazy {
StdDelegatingDeserializer(this)
}
}
// S is nullable because value corresponds to a nullable value class
// @see KotlinNamesAnnotationIntrospector.findNullSerializer
internal class ValueClassBoxConverter(
unboxedClass: Class,
val boxedClass: KClass
) : StdConverter() {
private val boxMethod = boxedClass.java.getDeclaredMethod("box-impl", unboxedClass).apply {
if (!this.isAccessible) this.isAccessible = true
}
@Suppress("UNCHECKED_CAST")
override fun convert(value: S): D = boxMethod.invoke(null, value) as D
val delegatingSerializer: StdDelegatingSerializer by lazy { StdDelegatingSerializer(this) }
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy