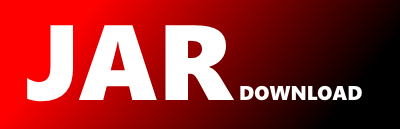
com.fathzer.soft.ajlib.swing.widget.RotatingLabel Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ajlib Show documentation
Show all versions of ajlib Show documentation
AJlib is a simple java library with Swing widgets, utilities and other stuff
package com.fathzer.soft.ajlib.swing.widget;
import java.awt.Dimension;
import java.awt.Graphics;
import java.awt.Graphics2D;
import java.awt.geom.AffineTransform;
import javax.swing.JLabel;
/** A JLabel that can be rotated.
* @author Jean-Marc Astesana
*
License: LGPL v3
*/
public class RotatingLabel extends JLabel {
private static final long serialVersionUID = 1L;
private int angle;
private boolean drawing;
/** Constructor.
*
By default, the label contains no text, no icon and is not rotated.
*/
public RotatingLabel() {
this.angle = 0;
}
/** Sets the angle of this label.
* @param angleDegrees The angle in degrees.
* @throws UnsupportedOperationException In the current version, only -90, 0, 90 and 180 are accepted. Other values throw this exception
*/
public void setRotation(int angleDegrees) {
angleDegrees = angleDegrees % 360;
if (angleDegrees < 0) {
angleDegrees = 360 + angleDegrees;
}
if (angleDegrees % 90 !=0) {
throw new UnsupportedOperationException();
}
angle = angleDegrees;
revalidate();
repaint();
}
@Override
public Dimension getPreferredSize() {
Dimension preferredSize = super.getPreferredSize();
double rad = Math.toRadians(angle);
int w = (int) (Math.abs(preferredSize.width*Math.cos(rad)) + Math.abs(preferredSize.height*Math.sin(rad)));
int h = (int) (Math.abs(preferredSize.width*Math.sin(rad)) + Math.abs(preferredSize.height*Math.cos(rad)));
Dimension result = new Dimension(w, h);
// System.out.println ("Angle: "+angle+", original pref size: "+preferredSize+" ,rotated: "+result);
return result;
}
@Override
public Dimension getSize() {
Dimension size = super.getSize();
if (!drawing) {
return size;
} else {
double rad = Math.toRadians(angle);
int w = (int) (Math.abs(size.width*Math.cos(rad)) + Math.abs(size.height*Math.sin(rad)));
int h = (int) (Math.abs(size.width*Math.sin(rad)) + Math.abs(size.height*Math.cos(rad)));
return new Dimension(w, h);
}
}
@Override
public int getHeight() {
return getSize().height;
}
@Override
public int getWidth() {
return getSize().width;
}
@Override
protected void paintComponent(Graphics g) {
Graphics2D gr = (Graphics2D) g.create();
if (angle==90) {
gr.transform(AffineTransform.getQuadrantRotateInstance(1));
gr.translate(0, -getSize().getWidth());
} else if (angle==270) {
gr.translate(0, getSize().getHeight());
gr.transform(AffineTransform.getQuadrantRotateInstance(3));
} else if (angle==180) {
gr.transform(AffineTransform.getQuadrantRotateInstance(2, (double)getWidth()/2, (double)getHeight()/2));
} else if (angle!=0) {
gr.transform(AffineTransform.getRotateInstance(Math.toRadians(angle), (double)getWidth()/2, (double)getHeight()/2));
// gr.translate(Math.cos(angle)*getSize().getWidth(), Math.sin(angle)*getSize().getHeight());
}
this.drawing = true;
super.paintComponent(gr);
this.drawing = false;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy