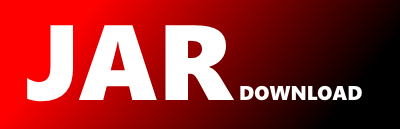
com.faunadb.client.HttpResponses Maven / Gradle / Ivy
package com.faunadb.client;
import com.fasterxml.jackson.core.JsonParseException;
import com.fasterxml.jackson.core.JsonParser;
import com.fasterxml.jackson.databind.DeserializationContext;
import com.fasterxml.jackson.databind.JsonDeserializer;
import com.fasterxml.jackson.databind.JsonNode;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.fasterxml.jackson.databind.annotation.JsonDeserialize;
import com.fasterxml.jackson.databind.type.TypeFactory;
import java.io.IOException;
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
public class HttpResponses {
static class Codec {
static class ValidationFailureDeserializer extends JsonDeserializer {
@Override
public ValidationFailure deserialize(JsonParser jsonParser, DeserializationContext deserializationContext) throws IOException {
ObjectMapper json = (ObjectMapper) jsonParser.getCodec();
TypeFactory tf = deserializationContext.getTypeFactory();
JsonNode tree = json.readTree(jsonParser);
if (!tree.has("field")) {
throw new JsonParseException(jsonParser, "Cannot deserialize ValidationFailure: no 'field' field.", jsonParser.getTokenLocation());
}
List field = json.convertValue(tree.get("field"), tf.constructCollectionType(ArrayList.class, String.class));
if (!tree.has("code")) {
throw new JsonParseException(jsonParser, "Cannot deserialize ValidationFailure: no 'code' field.", jsonParser.getTokenLocation());
}
String code = tree.get("code").asText();
String description;
if (tree.has("description")) {
description = tree.get("description").asText();
} else if (tree.has("reason")) {
description = tree.get("reason").asText();
} else {
throw new JsonParseException(jsonParser, "Cannot deserialize ValidationFailure: no 'description' field.", jsonParser.getTokenLocation());
}
return new ValidationFailure(field, code, description);
}
}
static class QueryErrorDeserializer extends JsonDeserializer {
@Override
public QueryError deserialize(JsonParser jsonParser, DeserializationContext deserializationContext) throws IOException {
ObjectMapper json = (ObjectMapper) jsonParser.getCodec();
JsonNode tree = json.readTree(jsonParser);
TypeFactory tf = deserializationContext.getTypeFactory();
List position;
if (tree.has("position")) {
position = json.convertValue(tree.get("position"), tf.constructCollectionType(ArrayList.class, String.class));
} else {
position = Collections.emptyList();
}
if (!tree.has("code")) {
throw new JsonParseException(jsonParser, "Cannot deserialize QueryError: no 'code' field.", jsonParser.getTokenLocation());
}
String code = tree.get("code").asText();
String description;
if (tree.has("description")) {
description = tree.get("description").asText();
} else {
throw new JsonParseException(jsonParser, "Cannot deserialize QueryError: no 'description' field.", jsonParser.getTokenLocation());
}
List failures;
if (tree.has("failures")) {
failures = json.convertValue(tree.get("failures"), tf.constructCollectionType(ArrayList.class, ValidationFailure.class));
} else {
failures = Collections.emptyList();
}
List cause;
if (tree.has("cause")) {
cause = json.convertValue(tree.get("cause"), tf.constructCollectionType(ArrayList.class, QueryError.class));
} else {
cause = Collections.emptyList();
}
return new QueryError(position, code, description, failures, cause);
}
}
}
@JsonDeserialize(using = Codec.ValidationFailureDeserializer.class)
public static class ValidationFailure {
private final List field;
private final String code;
private final String description;
public ValidationFailure(
List field,
String code,
String description) {
this.field = Collections.unmodifiableList(field);
this.code = code;
this.description = description;
}
public String code() {
return code;
}
public String description() {
return description;
}
public List field() {
return field;
}
}
@JsonDeserialize(using = Codec.QueryErrorDeserializer.class)
public static class QueryError {
private final List position;
private final String code;
private final String description;
private final List failures;
private final List cause;
public QueryError(List position,
String code,
String description,
List failures,
List cause) {
this.position = Collections.unmodifiableList(position);
this.code = code;
this.description = description;
this.failures = Collections.unmodifiableList(failures);
this.cause = Collections.unmodifiableList(cause);
}
public List position() {
return position;
}
public String code() {
return code;
}
public String description() {
return description;
}
public List failures() {
return failures;
}
public List getCause() {
return cause;
}
}
public static class QueryErrorResponse {
public static QueryErrorResponse create(int status, List errors) {
return new QueryErrorResponse(status, Collections.unmodifiableList(errors));
}
private final int status;
private final List errors;
public QueryErrorResponse(int status, List errors) {
this.status = status;
this.errors = errors;
}
public int status() {
return status;
}
public List errors() {
return errors;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy