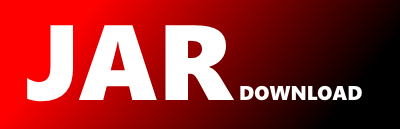
com.feedzai.fos.server.remote.api.FOSManagerAdapter Maven / Gradle / Ivy
/*
* $#
* FOS API
*
* Copyright (C) 2013 Feedzai SA
*
* This software is licensed under the Apache License, Version 2.0 (the "Apache License") or the GNU
* Lesser General Public License version 3 (the "GPL License"). You may choose either license to govern
* your use of this software only upon the condition that you accept all of the terms of either the Apache
* License or the LGPL License.
*
* You may obtain a copy of the Apache License and the LGPL License at:
*
* http://www.apache.org/licenses/LICENSE-2.0.txt
* http://www.gnu.org/licenses/lgpl-3.0.txt
*
* Unless required by applicable law or agreed to in writing, software distributed under the Apache License
* or the LGPL License is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND,
* either express or implied. See the Apache License and the LGPL License for the specific language governing
* permissions and limitations under the Apache License and the LGPL License.
* #$
*/
package com.feedzai.fos.server.remote.api;
import com.feedzai.fos.api.*;
import com.feedzai.fos.common.validation.NotBlank;
import java.rmi.NotBoundException;
import java.rmi.RemoteException;
import java.rmi.registry.LocateRegistry;
import java.rmi.registry.Registry;
import java.util.List;
import java.util.Map;
import java.util.UUID;
/**
* FOS Remote manager local adapter.
*
* @author Miguel Duarte ([email protected])
* @since 0.3.0
* @since 0.3.0
*/
public class FOSManagerAdapter implements Manager {
private final KryoScorer kryoScorer;
IRemoteManager manager;
public FOSManagerAdapter(IRemoteManager manager, KryoScorer kryoScorer) {
this.manager = manager;
this.kryoScorer = kryoScorer;
}
public UUID addModel(ModelConfig modelConfig, Model binary) throws FOSException {
try {
return manager.addModel(modelConfig, binary);
} catch (RemoteException e) {
throw new FOSException(e);
}
}
public UUID addModel(ModelConfig modelConfig, @NotBlank ModelDescriptor descriptor) throws FOSException {
try {
return manager.addModel(modelConfig, descriptor);
} catch (RemoteException e) {
throw new FOSException(e);
}
}
public void removeModel(UUID uuid) throws FOSException {
try {
manager.removeModel(uuid);
} catch (RemoteException e) {
throw new FOSException(e);
}
}
public void reconfigureModel(UUID uuid, ModelConfig modelConfig) throws FOSException {
try {
manager.reconfigureModel(uuid, modelConfig);
} catch (RemoteException e) {
throw new FOSException(e);
}
}
public void reconfigureModel(UUID uuid, ModelConfig modelConfig, Model bytes) throws FOSException {
try {
manager.reconfigureModel(uuid, modelConfig, bytes);
} catch (RemoteException e) {
throw new FOSException(e);
}
}
public void reconfigureModel(UUID uuid, ModelConfig modelConfig, @NotBlank ModelDescriptor descriptor) throws FOSException {
try {
manager.reconfigureModel(uuid, modelConfig, descriptor);
} catch (RemoteException e) {
throw new FOSException(e);
}
}
public Map listModels() throws FOSException {
try {
return manager.listModels();
} catch (RemoteException e) {
throw new FOSException(e);
}
}
public Scorer getScorer() throws FOSException {
try {
if (kryoScorer != null) {
return kryoScorer;
} else {
return new FOSScorerAdapter(manager.getScorer());
}
} catch (RemoteException e) {
throw new FOSException(e);
}
}
public UUID trainAndAdd(ModelConfig modelConfig, List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy