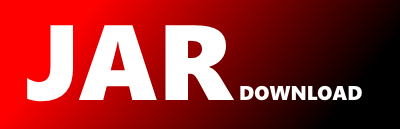
com.feedzai.fos.impl.weka.config.WekaModelConfig Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of fos-impl-weka Show documentation
Show all versions of fos-impl-weka Show documentation
Feedzai Open Scoring Server - Weka Implementation
/*
* $#
* FOS Weka
*
* Copyright (C) 2013 Feedzai SA
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public
* License along with this program. If not, see
* .
* #$
*/
package com.feedzai.fos.impl.weka.config;
import com.feedzai.fos.api.Attribute;
import com.feedzai.fos.api.FOSException;
import com.feedzai.fos.api.ModelConfig;
import com.feedzai.fos.common.validation.NotNull;
import com.feedzai.fos.impl.weka.utils.pool.GenericObjectPoolConfig;
import org.apache.commons.configuration.Configuration;
import org.apache.commons.configuration.ConfigurationConverter;
import org.apache.commons.configuration.MapConfiguration;
import java.io.File;
import java.util.List;
import java.util.Map;
import java.util.UUID;
import static com.google.common.base.Preconditions.checkNotNull;
/**
* Represents header that defines the schema of the machine learning model.
*
* @author Marco Jorge ([email protected])
*/
public class WekaModelConfig {
/**
* The property name of the classIndex.
* The classIndex is an integer that indicates the position of the classified instance fields (0 based).
*/
public static final String CLASS_INDEX = "classIndex";
/**
* The property name of the model file.
* The model file is the serialized Classifier that will be used for classification.
*/
public static final String MODEL_FILE = "model";
/**
* The property name of a boolean that indicates if the classifier is thread safe..
* If the model is thread safe then @{WekaThreadSafeScorerPassthrough} will be used, else @{WekaThreadSafeScorerPool} will be used.
*/
public static final String IS_CLASSIFIER_THREAD_SAFE = "isClassifierThreadSafe";
/**
* The property name that defines the weka classifier class (FQCN).
*/
public static final String CLASSIFIER_IMPL = "classifierimpl";
/**
* The property name of the ID of the model.
*/
public static final String ID = "id";
/**
* The property name of the model configuration.
*/
public static final String CLASSIFIER_CONFIG = "config";
private ModelConfig modelConfig;
private WekaManagerConfig wekaManagerConfig;
private int classIndex;
private File model;
private UUID id;
private transient File header;
private transient boolean dirty = true;
private boolean classifierThreadSafe;
private Configuration configuration;
/**
* Creates a new model from the given ModelConfig
and WekaManagerConfig
.
*
* From the ModelConfig.properties
the parameters MODEL_FILE
, ID
and CLASS_INDEX
are looked up.
* If the CLASS_INDEX
doesn't exist int he ModelConfig
, the default value is used from WekaManagerConfig
.
*
* @param modelConfig the configuration with MODEL_FILE
, ID
and CLASS_INDEX
* @param wekaManagerConfig the configuration with the default CLASS_INDEX
*/
public WekaModelConfig(ModelConfig modelConfig, WekaManagerConfig wekaManagerConfig) throws FOSException {
checkNotNull(modelConfig, "Model configuration cannot be null");
checkNotNull(wekaManagerConfig, "Manager configuration cannot be null");
this.modelConfig = modelConfig;
this.wekaManagerConfig = wekaManagerConfig;
parseModelConfig();
}
private void parseModelConfig() throws FOSException {
configuration = new MapConfiguration((Map) modelConfig.getProperties());
classIndex = this.modelConfig.getIntProperty(CLASS_INDEX, -1);
if (classIndex < 0) {
classIndex = this.modelConfig.getAttributes().size() - 1;
}
String modelFile = configuration.getString(MODEL_FILE);
if (modelFile != null) {
this.model = new File(modelFile);
}
classifierThreadSafe = configuration.getBoolean(IS_CLASSIFIER_THREAD_SAFE, false /* defaults to Pool implementation*/);
String uuid = configuration.getString(ID);
if (uuid != null) {
this.id = UUID.fromString(uuid);
}
}
/**
* Returns the pool configuration of the scorer.
*
* @return a map from configuration key to configuration value
*/
@NotNull
public Map
© 2015 - 2025 Weber Informatics LLC | Privacy Policy