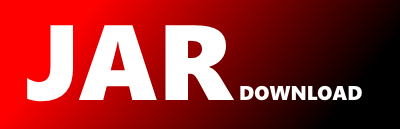
com.feingto.cloud.config.annotation.ApplicationContextHold Maven / Gradle / Ivy
The newest version!
package com.feingto.cloud.config.annotation;
import com.feingto.cloud.kit.CastUtils;
import lombok.extern.slf4j.Slf4j;
import org.springframework.beans.BeansException;
import org.springframework.beans.factory.config.BeanDefinition;
import org.springframework.beans.factory.support.BeanDefinitionRegistry;
import org.springframework.context.ApplicationContext;
import org.springframework.context.ApplicationContextAware;
import org.springframework.context.ApplicationContextException;
import org.springframework.core.Ordered;
import org.springframework.core.PriorityOrdered;
import org.springframework.lang.NonNull;
import java.lang.annotation.Annotation;
import java.util.Map;
/**
* 应用上下文
*
* @author longfei
*/
@Slf4j
public class ApplicationContextHold implements ApplicationContextAware, PriorityOrdered {
private static ApplicationContext ctx;
@Override
public void setApplicationContext(@NonNull ApplicationContext applicationContext) throws BeansException {
ApplicationContextHold.ctx = applicationContext;
}
@Override
public int getOrder() {
return Ordered.HIGHEST_PRECEDENCE + 1;
}
public static ApplicationContext getApplicationContext() {
checkApplicationContext();
return ctx;
}
public static T getBean(String beanName) {
try {
return CastUtils.cast(ctx.getBean(beanName));
} catch (Exception e) {
log.error("Consider defining a bean of name '{}' in your configuration.", beanName);
return null;
}
}
public static T getBean(Class requiredType) {
try {
return ctx.getBean(requiredType);
} catch (Exception e) {
log.error("Consider defining a bean of type '{}' in your configuration.", requiredType.getName());
return null;
}
}
public static Map getBeansOfType(Class requiredType) {
try {
return ctx.getBeansOfType(requiredType);
} catch (Exception e) {
log.error("Consider defining a bean of type '{}' in your configuration.", requiredType.getName());
return null;
}
}
public static String[] getBeanNamesForAnnotation(Class extends Annotation> annotationType) {
try {
return ctx.getBeanNamesForAnnotation(annotationType);
} catch (Exception e) {
log.error("Consider defining a bean of annotation type '{}' in your configuration.", annotationType.getName());
return null;
}
}
public static Map getBeansWithAnnotation(Class extends Annotation> annotationType) {
try {
return ctx.getBeansWithAnnotation(annotationType);
} catch (Exception e) {
log.error("Consider defining a bean of annotation type '{}' in your configuration.", annotationType.getName());
return null;
}
}
public static T createBean(Class requiredType) {
try {
return getApplicationContext().getAutowireCapableBeanFactory().createBean(requiredType);
} catch (Exception e) {
log.error("Consider defining a bean of type '{}' in your configuration.", requiredType.getName());
return null;
}
}
public static void registerBean(String beanName, BeanDefinition beanDefinition) {
CastUtils.cast(getApplicationContext().getAutowireCapableBeanFactory())
.registerBeanDefinition(beanName, beanDefinition);
}
public static void removeBean(String beanName) {
CastUtils.cast(getApplicationContext().getAutowireCapableBeanFactory())
.removeBeanDefinition(beanName);
}
private static void checkApplicationContext() {
if (ctx == null) {
throw new ApplicationContextException("Invalid application context: needs to be of type [" + ApplicationContextHold.class.getName() + "]");
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy