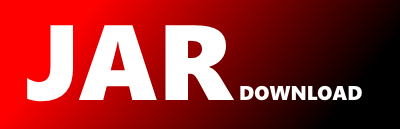
com.feingto.cloud.config.redis.adapter.RedisCacheableAroundAdvice Maven / Gradle / Ivy
The newest version!
package com.feingto.cloud.config.redis.adapter;
import com.feingto.cloud.config.redis.RedisCacheable;
import com.feingto.cloud.config.redis.support.CacheUtils;
import com.feingto.cloud.config.redis.support.SpELContext;
import com.feingto.cloud.kit.json.JSON;
import lombok.extern.slf4j.Slf4j;
import org.aopalliance.intercept.MethodInterceptor;
import org.aopalliance.intercept.MethodInvocation;
import org.springframework.data.redis.core.BoundValueOperations;
import org.springframework.data.redis.core.RedisTemplate;
import org.springframework.util.StringUtils;
import java.lang.reflect.Method;
/**
* RedisCacheable 环绕通知代理
*
* @author longfei
*/
@Slf4j
public class RedisCacheableAroundAdvice implements MethodInterceptor {
private final RedisTemplate redisTemplate;
public RedisCacheableAroundAdvice(RedisTemplate redisTemplate) {
this.redisTemplate = redisTemplate;
}
@Override
public Object invoke(MethodInvocation invocation) throws Throwable {
Method method = invocation.getMethod();
Object[] args = invocation.getArguments();
SpELContext.putContext(this.getClass(), method, args);
RedisCacheable cacheable = method.getAnnotation(RedisCacheable.class);
String cacheName = StringUtils.hasLength(cacheable.cacheName())
? cacheable.cacheName()
: CacheUtils.generateName(invocation.getThis());
String key = StringUtils.hasLength(cacheable.key())
? SpELContext.parserEl(cacheable.key())
: CacheUtils.generateKey(method, args);
BoundValueOperations opts = redisTemplate.boundValueOps(cacheName + ":" + key);
String value = opts.get();
Object result;
if (StringUtils.hasText(value)) {
log.trace("Cache >>> get key >>> {}", key);
Class> clazz = cacheable.clz();
try {
result = JSON.json2pojo(value, clazz);
} catch (Exception e) {
log.trace(e.getMessage());
try {
result = JSON.json2list(value, clazz);
} catch (Exception ex) {
log.error(e.getMessage());
result = invocation.proceed();
}
}
} else {
result = invocation.proceed();
log.trace("Cache >>> put key >>> {}", key);
opts.set(JSON.obj2json(result));
long expire = cacheable.expire();
if (expire > 0) {
opts.expire(expire, cacheable.timeUnit());
}
}
SpELContext.removeContext();
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy