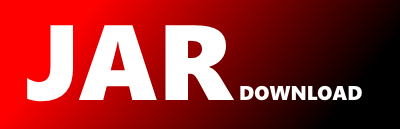
com.feingto.cloud.data.elasticsearch.BaseService Maven / Gradle / Ivy
package com.feingto.cloud.data.elasticsearch;
import com.feingto.cloud.data.bean.Page;
import com.feingto.cloud.kit.CastUtils;
import com.feingto.cloud.kit.reflection.ReflectionKit;
import org.elasticsearch.index.query.QueryBuilder;
import org.elasticsearch.search.sort.ScoreSortBuilder;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.data.elasticsearch.core.*;
import org.springframework.data.elasticsearch.core.mapping.IndexCoordinates;
import org.springframework.data.elasticsearch.core.query.NativeSearchQuery;
import org.springframework.data.elasticsearch.core.query.NativeSearchQueryBuilder;
import org.springframework.stereotype.Service;
import org.springframework.transaction.annotation.Transactional;
import org.springframework.util.Assert;
import java.io.Serializable;
import java.util.List;
import java.util.Optional;
import java.util.stream.Collectors;
/**
* ElasticSearch Crud 通用接口实现
*
* @author longfei
*/
@Service
@Transactional(readOnly = true, rollbackFor = Exception.class)
public abstract class BaseService implements IBase {
protected final Class clazz;
@Autowired
private ElasticsearchOperations operations;
public BaseService() {
this.clazz = ReflectionKit.getClassGenricType(getClass());
}
@Override
@Transactional(rollbackFor = Exception.class)
public T save(T entity) {
return operations.save(entity);
}
@Override
@Transactional(rollbackFor = Exception.class)
public void updateByProperty(ID id, String property, Object value) {
Assert.notNull(id, "Id is required");
Assert.notNull(property, "Property is required");
Optional.ofNullable(operations.get(stringIdRepresentation(id), clazz, getIndexCoordinates()))
.ifPresent(optional -> {
ReflectionKit.setFieldValue(optional, property, value);
operations.save(optional);
});
}
@Override
@Transactional(rollbackFor = Exception.class)
public void delete(ID id) {
Assert.notNull(id, "Id is required");
operations.delete(stringIdRepresentation(id), clazz);
}
@Override
public long count(QueryBuilder queryBuilder) {
NativeSearchQuery searchQuery = new NativeSearchQueryBuilder()
.withQuery(queryBuilder)
.build();
return operations.count(searchQuery, clazz, getIndexCoordinates());
}
@Override
public T findById(ID id) {
Assert.notNull(id, "Id is required");
return operations.get(stringIdRepresentation(id), clazz, getIndexCoordinates());
}
@Override
public List findAll(QueryBuilder queryBuilder) {
NativeSearchQuery searchQuery = new NativeSearchQueryBuilder()
.withQuery(queryBuilder)
.withSort(new ScoreSortBuilder())
.build();
return findAll(searchQuery);
}
@Override
public List findAll(NativeSearchQuery searchQuery) {
SearchHits searchHits = operations.search(searchQuery, clazz, getIndexCoordinates());
return searchHits
.getSearchHits().stream()
.map(SearchHit::getContent)
.collect(Collectors.toList());
}
@Override
public Page findByPage(QueryBuilder queryBuilder, Page page) {
NativeSearchQuery searchQuery = new NativeSearchQueryBuilder()
.withQuery(queryBuilder)
.withSort(new ScoreSortBuilder())
.build();
return findByPage(searchQuery, page);
}
@Override
public Page findByPage(NativeSearchQuery searchQuery, Page page) {
searchQuery.setPageable(Page.createPageable(page));
SearchHits searchHits = operations.search(searchQuery, clazz, getIndexCoordinates());
SearchPage searchPage = SearchHitSupport.searchPageFor(searchHits, searchQuery.getPageable());
Page.injectPageProperties(page, CastUtils.cast(searchPage));
return page.setContent(searchPage.getContent().stream()
.map(SearchHit::getContent)
.collect(Collectors.toList()));
}
private String stringIdRepresentation(ID id) {
return operations.stringIdRepresentation(id);
}
protected IndexCoordinates getIndexCoordinates() {
return operations.getIndexCoordinatesFor(clazz);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy