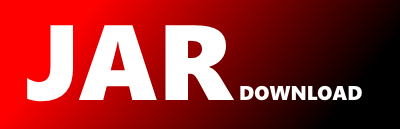
com.feingto.cloud.cache.RedisManager Maven / Gradle / Ivy
package com.feingto.cloud.cache;
import lombok.Getter;
import lombok.Setter;
import lombok.experimental.Accessors;
import org.springframework.data.redis.connection.RedisConnectionCommands;
import org.springframework.data.redis.core.*;
/**
* Redis 缓存管理器
*
* @author longfei
*/
@Accessors(chain = true)
public class RedisManager {
@Getter
@Setter
private RedisTemplate template;
public ValueOperations getValueStore() {
return template.opsForValue();
}
public HashOperations getHashStore() {
return template.opsForHash();
}
public ListOperations getListStore() {
return template.opsForList();
}
public SetOperations getSetStore() {
return template.opsForSet();
}
public ZSetOperations getZSetStore() {
return template.opsForZSet();
}
public ClusterOperations getClusterStore() {
return template.opsForCluster();
}
public HyperLogLogOperations getHyperLogLogStore() {
return template.opsForHyperLogLog();
}
public String getPing() {
return (String) template.execute((RedisCallback
© 2015 - 2025 Weber Informatics LLC | Privacy Policy