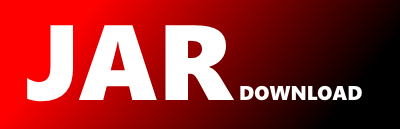
com.feingto.cloud.cache.provider.RedisHashCache Maven / Gradle / Ivy
package com.feingto.cloud.cache.provider;
import com.feingto.cloud.cache.RedisManager;
import com.feingto.cloud.kit.json.JSON;
import org.springframework.data.redis.core.BoundHashOperations;
import org.springframework.data.redis.core.RedisTemplate;
import java.util.*;
import java.util.concurrent.TimeUnit;
import java.util.stream.Collectors;
/**
* Redis 哈希缓存操作
*
* @author longfei
*/
@SuppressWarnings("unchecked")
public class RedisHashCache {
private static RedisManager redisManager;
public RedisHashCache(RedisTemplate template) {
redisManager = new RedisManager().setTemplate(template);
}
public T deserialize(String json) {
return this.deserialize(json, Object.class);
}
public T deserialize(String json, Class clazz) {
return (T) JSON.json2pojo(json, clazz);
}
/**
* 获取指定key的Map值
*
* @param key key
*/
public Map get(String key) {
return this.get(key, Object.class);
}
/**
* 获取指定key的Map值
*
* @param key key
* @param clazz 对象类
*/
public Map get(String key, Class clazz) {
return Optional.ofNullable(redisManager.getHashStore().getOperations().boundHashOps(key).entries())
.map(map -> map.entrySet().stream().collect(Collectors.toMap(entry ->
(String) entry.getKey(), entry -> this.deserialize((String) entry.getValue(), clazz))))
.orElse(new HashMap<>());
}
/**
* 获取指定key中field的值
*
* @param key key
* @param field field
*/
public T get(String key, String field) {
return this.get(key, field, Object.class);
}
/**
* 获取指定key中field的对象值
*
* @param key key
* @param field field
* @param clazz 对象类
*/
public T get(String key, String field, Class clazz) {
return deserialize((String) redisManager.getHashStore().getOperations().boundHashOps(key).get(field), clazz);
}
/**
* 获取指定key中field的列表值
*
* @param key key
* @param field field
*/
public List getList(String key, String field, Class clazz) {
return JSON.json2list((String) redisManager.getHashStore()
.getOperations().boundHashOps(key).get(field), clazz);
}
/**
* 获取指定key中field的Map值
*
* @param key key
* @param field field
*/
public Map getMap(String key, String field, Class clazz) {
return JSON.json2map((String) redisManager.getHashStore()
.getOperations().boundHashOps(key).get(field), clazz);
}
/**
* 设置键值有效期
*
* @param key key
* @param field field
* @param value 值
*/
public void put(String key, String field, Object value) {
redisManager.getHashStore().getOperations().boundHashOps(key).put(field, JSON.obj2json(value));
}
/**
* 设置键值有效期
*
* @param key key
* @param field field
* @param value 值
* @param expireTime 过期时间,单位毫秒
*/
public void put(String key, String field, Object value, long expireTime) {
this.put(key, field, value, expireTime, TimeUnit.MILLISECONDS);
}
/**
* 设置键值有效期
*
* @param key key
* @param field field
* @param value 值
* @param expireTime 过期时间
* @param timeUnit 过期时间单位
*/
public void put(String key, String field, Object value, long expireTime, TimeUnit timeUnit) {
BoundHashOperations operations = redisManager.getHashStore().getOperations().boundHashOps(key);
operations.put(field, JSON.obj2json(value));
operations.expire(expireTime, timeUnit);
}
/**
* 获取缓存所有键
*
* @param key key
*/
public Set keys(String key) {
return Optional.ofNullable(redisManager.getHashStore().getOperations().boundHashOps(key).keys())
.map(keys -> keys.stream()
.map(k -> (String) k)
.collect(Collectors.toSet()))
.orElse(new HashSet<>());
}
/**
* 是否存在
*
* @param key key
* @param field field
*/
public boolean has(String key, String field) {
return Optional.ofNullable(redisManager.getHashStore().getOperations().boundHashOps(key).hasKey(field))
.orElse(false);
}
/**
* 移除
*
* @param key key
* @param field field
*/
public void remove(String key, String field) {
redisManager.getHashStore().getOperations().boundHashOps(key).delete(field);
}
/**
* 根据前缀移除
*
* @param key key
* @param prefix 前缀
*/
public void removeByPrefix(String key, String prefix) {
Optional.ofNullable(redisManager.getHashStore().getOperations().boundHashOps(key).keys())
.ifPresent(keys -> keys.forEach(k -> removeByPrefix(key, prefix + "*")));
}
/**
* 清空缓存
*
* @param key key
*/
public void clear(String key) {
redisManager.getHashStore().getOperations().delete(key);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy