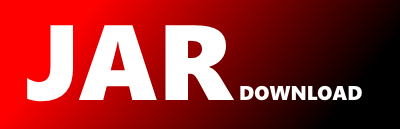
com.feingto.cloud.cache.provider.RedisLock Maven / Gradle / Ivy
package com.feingto.cloud.cache.provider;
import org.springframework.data.redis.core.RedisTemplate;
import org.springframework.data.redis.core.ValueOperations;
import org.springframework.util.StringUtils;
import java.util.Objects;
import java.util.concurrent.TimeUnit;
/**
* Redis 分布式锁
*
* @author longfei
*/
public class RedisLock {
private final ValueOperations operations;
public RedisLock(RedisTemplate redisTemplate) {
this.operations = redisTemplate.opsForValue();
}
/**
* 加锁
*
* @param key 键
* @param lockValue 当前锁值
*/
public boolean lock(String key, String lockValue) {
return this.lock(key, lockValue, null);
}
/**
* 加锁
*
* @param key 键
* @param lockValue 当前锁值
* @param lockSeconds 锁定秒数
*/
public boolean lock(String key, String lockValue, Integer lockSeconds) {
if (Objects.nonNull(lockSeconds) && operations.setIfAbsent(key, lockValue, lockSeconds, TimeUnit.SECONDS)) {
return true;
} else if (Objects.isNull(lockSeconds) && operations.setIfAbsent(key, lockValue)) {
return true;
}
String current = operations.get(key);
if (StringUtils.hasText(current) && Long.parseLong(current) < System.currentTimeMillis()) {
String old = operations.getAndSet(key, lockValue);
return StringUtils.hasText(old) && old.equals(current);
}
return false;
}
/**
* 解锁
*
* @param key 键
* @param lockValue 当前锁值
*/
public void unlock(String key, String lockValue) {
try {
String current = operations.get(key);
if (StringUtils.hasText(current) && current.equals(lockValue)) {
operations.getOperations().delete(key);
}
} catch (Exception ignored) {
}
}
/**
* 获取当前锁值
*
* @param expireTime 有效期秒数
* @return 当前时间毫秒数 + 超时时间毫秒数
*/
public String getLockValue(long expireTime) {
return String.valueOf(System.currentTimeMillis() + expireTime * 1000);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy