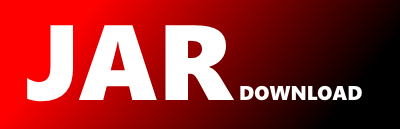
com.feingto.cloud.domain.account.Resource Maven / Gradle / Ivy
package com.feingto.cloud.domain.account;
import com.fasterxml.jackson.annotation.JsonIgnoreProperties;
import com.feingto.cloud.domain.BaseEntity;
import lombok.Data;
import lombok.EqualsAndHashCode;
import lombok.experimental.Accessors;
import org.apache.commons.collections.CollectionUtils;
import org.hibernate.annotations.ColumnDefault;
import org.hibernate.annotations.DynamicUpdate;
import org.hibernate.annotations.Fetch;
import org.hibernate.annotations.FetchMode;
import org.springframework.util.StringUtils;
import javax.persistence.*;
import javax.validation.constraints.NotBlank;
import java.util.ArrayList;
import java.util.List;
import java.util.Optional;
import java.util.stream.Collectors;
import java.util.stream.Stream;
/**
* 资源菜单
*
* @author longfei
*/
@Data
@EqualsAndHashCode(callSuper = true)
@Accessors(chain = true)
@Entity
@DynamicUpdate
@Table(name = "sy_res")
public class Resource extends BaseEntity {
private static final long serialVersionUID = -4096569750361322654L;
@NotBlank(message = "菜单名称不能为空")
@Column(nullable = false, length = 64)
private String name;
@Column(nullable = false, length = 32)
private String sn;
@Column(nullable = false, length = 32)
private String code;
@Column(nullable = false)
private Integer location;
@Column(length = 256)
private String description;
@Column(length = 64)
private String parentId;
@Column
@ColumnDefault("false")
private boolean hasChildren = false;
@Column
@ColumnDefault("true")
private boolean enabled = true;
@JsonIgnoreProperties(value = {"resources", "userRoles"}, allowSetters = true)
@Fetch(FetchMode.SUBSELECT)
@ManyToMany(cascade = CascadeType.REFRESH)
@JoinTable(name = "sy_role_res",
joinColumns = {@JoinColumn(name = "res_id")},
inverseJoinColumns = {@JoinColumn(name = "role_id")})
private List roles = new ArrayList<>();
@JsonIgnoreProperties(value = "resource", allowSetters = true)
@Fetch(FetchMode.SUBSELECT)
@OneToMany(mappedBy = "resource", cascade = CascadeType.ALL, orphanRemoval = true)
@OrderBy("location asc ")
private List buttons = new ArrayList<>();
@JsonIgnoreProperties(value = "resource", allowSetters = true)
@Fetch(FetchMode.SUBSELECT)
@OneToMany(mappedBy = "resource", cascade = CascadeType.ALL, orphanRemoval = true)
@OrderBy("location asc ")
private List columns = new ArrayList<>();
@Column(name = "apps", length = 256)
private String apps;
@Transient
private List children = new ArrayList<>();
@Transient
private List appList = new ArrayList<>();
public Resource setParentId(String parentId) {
this.parentId = StringUtils.isEmpty(parentId) ? "-1" : parentId;
return this;
}
public Resource setButtons(List buttons) {
Optional.ofNullable(buttons)
.map(c -> {
this.buttons.clear();
this.buttons.addAll(c);
this.buttons.forEach(item -> item.setResource(this));
return c;
})
.orElseGet(() -> this.buttons = null);
return this;
}
public Resource setColumns(List columns) {
Optional.ofNullable(columns)
.map(c -> {
this.columns.clear();
this.columns.addAll(c);
this.columns.forEach(item -> item.setResource(this));
return c;
})
.orElseGet(() -> this.columns = null);
return this;
}
public List getAppList() {
return StringUtils.hasText(this.apps)
? Stream.of(this.apps.split(",", -1)).collect(Collectors.toList())
: this.appList;
}
public void setAppList(List appList) {
this.appList = appList;
this.apps = CollectionUtils.isNotEmpty(appList) ? String.join(",", appList) : null;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy