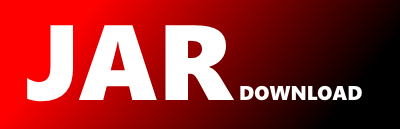
com.feingto.cloud.domain.account.User Maven / Gradle / Ivy
package com.feingto.cloud.domain.account;
import com.fasterxml.jackson.annotation.JsonIgnoreProperties;
import com.feingto.cloud.domain.BaseEntity;
import com.feingto.cloud.domain.enums.RoleType;
import com.feingto.cloud.domain.enums.SignType;
import lombok.Data;
import lombok.EqualsAndHashCode;
import lombok.experimental.Accessors;
import org.apache.commons.collections.CollectionUtils;
import org.hibernate.annotations.ColumnDefault;
import org.hibernate.annotations.DynamicUpdate;
import org.hibernate.annotations.Fetch;
import org.hibernate.annotations.FetchMode;
import org.springframework.util.StringUtils;
import javax.persistence.*;
import java.util.ArrayList;
import java.util.List;
import java.util.Objects;
import java.util.stream.Collectors;
/**
* 用户
*
* @author longfei
*/
@Data
@EqualsAndHashCode(callSuper = true)
@Accessors(chain = true)
@Entity
@DynamicUpdate
@Table(name = "sy_user")
public class User extends BaseEntity {
private static final long serialVersionUID = 4412527340716672096L;
public static final String SYSTEM_ADMIN = "admin";
public static final String ANONYMOUS_USER = "anonymousUser";
/**
* 第三方登录密码为空,反之密码必填
*/
@Column(length = 64)
private String password;
/**
* 姓名
*/
@Column(length = 64)
private String realName;
/**
* 身份证号
*/
@Column(length = 32)
private String identityCard;
/**
* 出生年月日
*/
@Column(length = 16)
private String birthday;
/**
* 页面布局
*/
@Column(length = 32)
private String layout;
/**
* 页面风格
*/
@Column(length = 32)
private String pageStyle;
/**
* 个人图像
*/
@Column
private String avatar;
@Column(nullable = false)
@ColumnDefault("true")
private boolean enabled = true;
@Fetch(FetchMode.SUBSELECT)
@OneToMany(mappedBy = "user", fetch = FetchType.EAGER, cascade = CascadeType.ALL, orphanRemoval = true)
private List userRoles = new ArrayList<>();
@Fetch(FetchMode.SUBSELECT)
@OneToMany(mappedBy = "user", fetch = FetchType.EAGER, cascade = CascadeType.ALL, orphanRemoval = true)
private List userAuths = new ArrayList<>();
@Transient
private String username;
@Transient
private String mobile;
@Transient
private String email;
@JsonIgnoreProperties(value = "roles", allowSetters = true)
@Transient
private List resources = new ArrayList<>();
@Transient
private List roleIds = new ArrayList<>();
private void addUserIdentifier(String identifier, SignType signType) {
this.userAuths.removeIf(userAuth -> signType.equals(userAuth.getSignType()));
if (StringUtils.hasText(identifier)) {
this.userAuths.add(new UserAuth()
.setSignType(signType)
.setIdentifier(identifier));
}
}
private String getUserIdentifier(SignType signType) {
return Objects.nonNull(this.userAuths) ? this.userAuths.stream()
.filter(userAuth -> signType.equals(userAuth.getSignType()))
.map(UserAuth::getIdentifier)
.findFirst()
.orElse(null) : null;
}
public void setUsername(String username) {
this.addUserIdentifier(username, SignType.USERNAME);
}
public String getUsername() {
return this.getUserIdentifier(SignType.USERNAME);
}
public void setMobile(String phone) {
this.addUserIdentifier(phone, SignType.MOBILE);
}
public String getMobile() {
return this.getUserIdentifier(SignType.MOBILE);
}
public void setEmail(String email) {
this.addUserIdentifier(email, SignType.EMAIL);
}
public String getEmail() {
return this.getUserIdentifier(SignType.EMAIL);
}
/**
* 是否超级管理员, 超级管理员不验证按钮、字段、数据权限
*/
public boolean isSystemAdmin() {
return CollectionUtils.isNotEmpty(this.userRoles) && this.userRoles.stream()
.map(UserRole::getRole)
.anyMatch(role -> RoleType.ADMIN.name().equals(role.getSn()));
}
public List getRoleIds() {
return CollectionUtils.isNotEmpty(this.userRoles) ? this.userRoles.stream()
.map(UserRole::getRole)
.map(Role::getId)
.collect(Collectors.toList()) : this.roleIds;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy