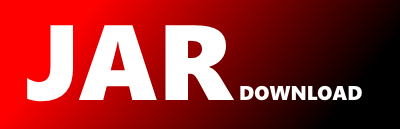
com.feingto.cloud.domain.api.BaseApi Maven / Gradle / Ivy
package com.feingto.cloud.domain.api;
import com.feingto.cloud.constants.Constants;
import com.feingto.cloud.constants.HttpConstants;
import com.feingto.cloud.domain.BaseEntity;
import com.feingto.cloud.domain.converters.ParameterPersistenceConverters;
import com.feingto.cloud.domain.enums.ParamMode;
import com.feingto.cloud.domain.enums.Stage;
import com.feingto.cloud.dto.apis.ParameterDTO;
import com.feingto.cloud.kit.KeyValue;
import lombok.Data;
import lombok.EqualsAndHashCode;
import lombok.experimental.Accessors;
import org.hibernate.annotations.ColumnDefault;
import org.springframework.http.HttpMethod;
import javax.persistence.*;
import javax.validation.constraints.NotBlank;
import java.util.ArrayList;
import java.util.List;
import java.util.Objects;
/**
* API 定义
*
* @author longfei
*/
@Data
@EqualsAndHashCode(callSuper = true)
@Accessors(chain = true)
@MappedSuperclass
public class BaseApi extends BaseEntity {
private static final long serialVersionUID = -4931656195007699107L;
@NotBlank(message = "请求Path不能为空")
@Column(nullable = false)
protected String path;
/**
* 请求方式
*/
@Enumerated(EnumType.STRING)
@Column(length = 16)
protected HttpMethod httpMethod;
/**
* 入参请求模式: 入参映射; 入参透传
*/
@Enumerated(EnumType.STRING)
@Column(length = 16)
@ColumnDefault("'PASSTHROUGH'")
protected ParamMode paramMode = ParamMode.PASSTHROUGH;
/**
* 是否自定义敏感Headers
*/
@Column
@ColumnDefault("false")
protected boolean customSensitiveHeaders = false;
/**
* 是否启用OAuth2授权验证
*/
@Column
@ColumnDefault("false")
protected boolean authorized = false;
/**
* 敏感Headers
*/
@Column
protected String sensitiveHeaders;
/**
* 请求参数
*/
@Convert(converter = ParameterPersistenceConverters.class)
@Column(name = "request_params", columnDefinition = "text")
protected List requestParams = new ArrayList<>();
/**
* 非Form表单数据,比如JSON字符串、文件二进制数据等
*/
@Column
@ColumnDefault("false")
protected boolean body = false;
/**
* 预期服务器返回的数据类型
*/
@Column
@ColumnDefault("'JSON'")
protected String contentType = "JSON";
/**
* 是否开启缓存
*/
@Column
@ColumnDefault("false")
protected boolean cacheable = false;
/**
* 缓存有效时间(单位:秒)
*/
@Column
@ColumnDefault("60")
protected Integer cachetime = Constants.DEFAULT_CACHE_TIMEOUT;
/**
* 是否使用Mock
*/
@Column
@ColumnDefault("false")
protected boolean mock = false;
/**
* 返回参数
*/
@Convert(converter = ParameterPersistenceConverters.class)
@Column(name = "response_params", columnDefinition = "text")
protected List responseParams = new ArrayList<>();
/**
* API 唯一标识(管理平台API的ID)
*/
@Transient
protected String sn;
/**
* API 名称
*/
@Transient
protected String name;
/**
* API 描述
*/
@Transient
private String description;
/**
* 分组ID
*/
@Transient
protected String groupId;
/**
* 分组名称
*/
@Transient
protected String groupName;
/**
* 分组流量限制(QPS)
*/
@Transient
protected Long groupQps = 0L;
/**
* 环境变量参数
*/
@Transient
protected List params = new ArrayList<>();
/**
* 环境(线上、预发、测试)
*/
@Transient
protected Stage stage;
/**
* API 路由
*/
@Transient
protected List baseApiRoutes = new ArrayList<>();
/**
* 熔断配置
*/
@Transient
protected BaseHystrix baseHystrix;
/**
* 流控策略
*/
@Transient
protected BaseStrategy baseStrategy;
/**
* 创建人
*/
@Transient
protected String owner;
public BaseApi setHttpMethod(HttpMethod httpMethod) {
this.httpMethod = Objects.isNull(httpMethod) || HttpConstants.API_HTTP_METHOD_ANY
.equalsIgnoreCase(httpMethod.name()) ? null : httpMethod;
return this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy