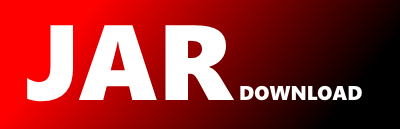
com.feingto.cloud.domain.api.BaseStrategy Maven / Gradle / Ivy
package com.feingto.cloud.domain.api;
import com.feingto.cloud.domain.BaseEntity;
import com.feingto.cloud.domain.enums.IntervalUnit;
import com.feingto.cloud.kit.json.JSON;
import lombok.Data;
import lombok.EqualsAndHashCode;
import lombok.experimental.Accessors;
import org.hibernate.annotations.ColumnDefault;
import org.springframework.integration.support.MessageBuilder;
import org.springframework.messaging.Message;
import javax.persistence.*;
import java.util.ArrayList;
import java.util.List;
/**
* 策略定义
*
* @author longfei
*/
@Data
@EqualsAndHashCode(callSuper = true)
@Accessors(chain = true)
@MappedSuperclass
public class BaseStrategy extends BaseEntity {
private static final long serialVersionUID = 7305595514377207094L;
public static final String API_FLOW_LIMIT = "API_FLOW_LIMIT";
public static final String API_EXCEPTION_ALARM = "API_EXCEPTION_ALARM";
public static final String API_TIMEOUT_ALARM = "API_TIMEOUT_ALARM";
/**
* 策略类型
*/
@Column
@ColumnDefault("'API_FLOW_LIMIT'")
protected String strategyType;
@Column
protected String name;
/**
* API流量限制次数
*/
@Column
@ColumnDefault("'0'")
protected Long apiLimit = 0L;
/**
* APP流量限制次数(不超过API流量限制值)
*/
@Column
@ColumnDefault("'0'")
protected Long appLimit = 0L;
/**
* 频率
*/
@Column
@ColumnDefault("'1'")
protected Long frequency = 1L;
/**
* 单位时间(分钟、小时、天)
*/
@Enumerated(EnumType.STRING)
@Column(length = 16)
@ColumnDefault("'MINUTES'")
protected IntervalUnit intervalUnit = IntervalUnit.MINUTES;
/**
* 阈值(监控值)
*/
@Column
protected String threshold;
/**
* 预警级别, 数据字典 rule_level
*/
@Column(name = "rule_level")
protected String level;
/**
* 邮箱地址(逗号连接字符串)
*/
@Column
protected String email;
/**
* 短信号码(逗号连接字符串)
*/
@Column
protected String sms;
/**
* 是否推送
*/
@Column
@ColumnDefault("false")
protected boolean push = false;
@Column(length = 512)
protected String description;
@Transient
protected List baseApis = new ArrayList<>();
public Message toMessage() {
return MessageBuilder.withPayload(JSON.obj2json(this)).build();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy