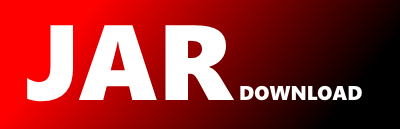
com.feingto.cloud.domain.system.Dict Maven / Gradle / Ivy
package com.feingto.cloud.domain.system;
import com.fasterxml.jackson.annotation.JsonIgnoreProperties;
import com.feingto.cloud.domain.BaseEntity;
import lombok.Data;
import lombok.EqualsAndHashCode;
import lombok.experimental.Accessors;
import org.hibernate.annotations.*;
import org.springframework.util.StringUtils;
import javax.persistence.CascadeType;
import javax.persistence.Entity;
import javax.persistence.OrderBy;
import javax.persistence.Table;
import javax.persistence.*;
import javax.validation.constraints.NotBlank;
import java.util.ArrayList;
import java.util.List;
import java.util.Optional;
/**
* 数据字典
*
* @author longfei
*/
@Data
@EqualsAndHashCode(callSuper = true)
@Accessors(chain = true)
@Entity
@Table(name = "sy_dict")
@DynamicUpdate
public class Dict extends BaseEntity {
private static final long serialVersionUID = -8461784632008392736L;
@Column(nullable = false)
private String code;
@NotBlank(message = "父节点不能为空")
@Column(nullable = false)
@ColumnDefault("'-1'")
private String parentId = "-1";
@Column
@ColumnDefault("false")
private boolean hasChildren = false;
@NotBlank(message = "标识不能为空")
@Column(nullable = false)
private String sn;
@Column
private String name;
@JsonIgnoreProperties(value = "dict", allowSetters = true)
@Fetch(FetchMode.SUBSELECT)
@OneToMany(mappedBy = "dict", cascade = CascadeType.ALL, orphanRemoval = true)
@OrderBy("location asc")
@Where(clause = "enabled=1")
private List items = new ArrayList<>();
@Transient
private List children = new ArrayList<>();
/**
* 默认为字典图标,字典项图标为:"fa fa-sitemap"
*/
@Transient
private String icon = "fa fa-folder";
public Dict setParentId(String parentId) {
this.parentId = StringUtils.isEmpty(parentId) ? "-1" : parentId;
return this;
}
public Dict setItems(List items) {
Optional.ofNullable(items)
.map(c -> {
this.items.clear();
this.items.addAll(c);
this.items.forEach(item -> item.setDict(this));
return c;
})
.orElseGet(() -> this.items = null);
return this;
}
/**
* 组装字典树
*/
public static List tree(List dictList) {
List dicts = new ArrayList<>();
dictList.forEach(dict -> {
if ("-1".equals(dict.getParentId())) {
dicts.add(dict);
}
dictList.stream()
.filter(child -> child.getParentId().equals(dict.getId()))
.forEach(child -> dict.getChildren().add(child));
});
return dicts;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy