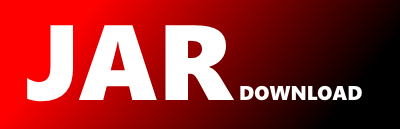
com.feingto.cloud.domain.account.Resource Maven / Gradle / Ivy
package com.feingto.cloud.domain.account;
import com.fasterxml.jackson.annotation.JsonIgnoreProperties;
import com.feingto.cloud.domain.BaseEntity;
import lombok.*;
import org.hibernate.annotations.*;
import javax.persistence.CascadeType;
import javax.persistence.*;
import javax.persistence.Entity;
import javax.persistence.OrderBy;
import javax.persistence.Table;
import java.util.ArrayList;
import java.util.Date;
import java.util.List;
/**
* 菜单资源
*
* @author longfei
*/
@Setter
@Getter
@NoArgsConstructor
@AllArgsConstructor(access = AccessLevel.PROTECTED)
@Entity
@DynamicUpdate
@Table(name = "sy_res")
public class Resource extends BaseEntity {
private static final long serialVersionUID = -4096569750361322654L;
@Column
protected String sn;
@Column
protected String name;
@Column
protected String code;
@Column
protected String url;
@Column
protected String icon;
@Column
protected Integer location;
@Builder.Default
@Column
@ColumnDefault("true")
protected boolean enabled = true;
@Column
protected String descript;
@Builder.Default
@Column
@ColumnDefault("false")
protected boolean hasChildren = false;
@Column
protected String parentId;
@Builder.Default
@Column
@ColumnDefault("false")
protected boolean beta = false;
@Builder.Default
@Column
@ColumnDefault("true")
protected boolean display = true;
@Builder.Default
@Column
@ColumnDefault("false")
protected boolean newWindow = false;
@Builder.Default
@JsonIgnoreProperties(value = {"resources", "userRoles"}, allowSetters = true)
@BatchSize(size = 30)
@Fetch(FetchMode.SELECT)
@ManyToMany(cascade = CascadeType.REFRESH)
@JoinTable(
name = "sy_role_res",
joinColumns = {@JoinColumn(name = "res_id")},
inverseJoinColumns = {@JoinColumn(name = "role_id")}
)
protected List roles = new ArrayList<>();
@Builder.Default
@JsonIgnoreProperties(value = "resource", allowSetters = true)
@BatchSize(size = 30)
@Fetch(FetchMode.SELECT)
@OneToMany(mappedBy = "resource", cascade = CascadeType.ALL, orphanRemoval = true)
@OrderBy("location asc ")
protected List columns = new ArrayList<>();
@Builder.Default
@JsonIgnoreProperties(value = "resource", allowSetters = true)
@BatchSize(size = 30)
@Fetch(FetchMode.SELECT)
@OneToMany(mappedBy = "resource", cascade = CascadeType.ALL, orphanRemoval = true)
@OrderBy("location asc ")
protected List buttons = new ArrayList<>();
@Transient
protected List children = new ArrayList<>();
@Builder
public Resource(String id, String createdBy, Date createdDate, String lastModifiedBy, Date lastModifiedDate, String sn, String name, String code, String url, String icon, Integer location, boolean enabled, String descript, boolean hasChildren, String parentId, boolean beta, boolean display, boolean newWindow, List roles, List columns, List buttons, List children) {
super(createdBy, createdDate, lastModifiedBy, lastModifiedDate);
this.id = id;
this.sn = sn;
this.name = name;
this.code = code;
this.url = url;
this.icon = icon;
this.location = location;
this.enabled = enabled;
this.descript = descript;
this.hasChildren = hasChildren;
this.parentId = parentId;
this.beta = beta;
this.display = display;
this.newWindow = newWindow;
this.roles = roles;
this.columns = columns;
this.buttons = buttons;
this.children = children;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy