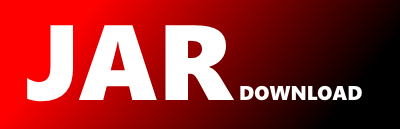
com.feingto.cloud.domain.account.User Maven / Gradle / Ivy
package com.feingto.cloud.domain.account;
import com.fasterxml.jackson.annotation.JsonIgnoreProperties;
import com.feingto.cloud.domain.BaseEntity;
import lombok.*;
import org.hibernate.annotations.*;
import org.springframework.util.CollectionUtils;
import javax.persistence.CascadeType;
import javax.persistence.*;
import javax.persistence.Entity;
import javax.persistence.Table;
import javax.validation.constraints.NotNull;
import javax.validation.constraints.Size;
import java.util.ArrayList;
import java.util.List;
import java.util.stream.Collectors;
/**
* 用户
*
* @author longfei
*/
@Builder
@Setter
@Getter
@NoArgsConstructor
@AllArgsConstructor(access = AccessLevel.PROTECTED)
@Entity
@DynamicUpdate
@Table(name = "sy_user")
public class User extends BaseEntity {
private static final long serialVersionUID = -7602047101257351821L;
public static final String SYSTEM_ADMIN = "admin";
@Size(max = 50)
@Column(length = 50, nullable = false, unique = true)
protected String username;
@Size(min = 1, max = 60)
@Column(length = 60, nullable = false)
protected String password;
@Column
protected String realName;
@Column
protected String pageStyle;
@Column
protected String layout;
@Column
protected String avatar;
@Builder.Default
@NotNull
@Column(nullable = false)
@ColumnDefault("true")
protected boolean enabled = true;
@Builder.Default
@BatchSize(size = 30)
@Fetch(FetchMode.SELECT)
@OneToMany(mappedBy = "user", fetch = FetchType.EAGER, cascade = CascadeType.ALL, orphanRemoval = true)
protected List userRoles = new ArrayList<>();
@JsonIgnoreProperties(value = "roles", allowSetters = true)
@Transient
protected List resources = new ArrayList<>();
@Builder.Default
@Transient
protected List roleIds = new ArrayList<>();
/**
* 是否超级管理员, 超级管理员不验证按钮、字段、数据权限
*/
public boolean isSystemAdmin() {
return SYSTEM_ADMIN.equals(this.username);
}
public List getRoleIds() {
return !CollectionUtils.isEmpty(this.userRoles) ? this.userRoles.stream().map(UserRole::getRole).map(Role::getId).collect(Collectors.toList()) : this.roleIds;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy