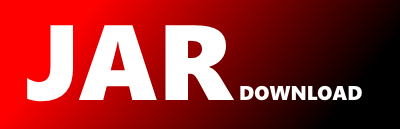
com.feingto.cloud.kit.SpringContextUtil Maven / Gradle / Ivy
package com.feingto.cloud.kit;
import org.springframework.beans.factory.NoSuchBeanDefinitionException;
import org.springframework.context.ApplicationContext;
import org.springframework.context.ApplicationContextAware;
import java.util.Map;
/**
* Spring Boot获取ApplicationContext
* 方式一: 与Spring Boot启动类同包或其子包,SpringContextUtil 加上 @Component
* 方式二:不同包, 在初始化过程中使用 @Bean 注入 SpringContextUtil 或者使用 @Import进行导入
*/
@SuppressWarnings("unused")
public class SpringContextUtil implements ApplicationContextAware {
private static ApplicationContext context = null;
/**
* 取得存储在静态变量中的ApplicationContext.
*/
public static ApplicationContext getApplicationContext() {
checkApplicationContext();
return context;
}
/**
* 实现ApplicationContextAware接口的context注入函数, 将其存入静态变量.
*/
public void setApplicationContext(ApplicationContext applicationContext) {
if (SpringContextUtil.context == null) {
context = applicationContext;
}
}
/**
* 从静态变量ApplicationContext中取得Bean, 自动转型为所赋值对象的类型.
*/
@SuppressWarnings("unchecked")
public static T getBean(String name) {
checkApplicationContext();
return (T) context.getBean(name);
}
/**
* 从静态变量ApplicationContext中取得Bean, 自动转型为所赋值对象的类型. 如果有多个Bean符合Class, 取出第一个.
*/
public static T getBean(Class clazz) {
checkApplicationContext();
Map beanMaps = context.getBeansOfType(clazz);
if (beanMaps != null && !beanMaps.isEmpty()) {
return beanMaps.values().iterator().next();
} else {
return null;
}
}
private static void checkApplicationContext() {
if (context == null) {
throw new IllegalStateException("ApplicaitonContext not injected, please define SpringContextUtil.");
}
}
public static boolean containsBean(String name) {
return context.containsBean(name);
}
public static boolean isSingleton(String name) throws NoSuchBeanDefinitionException {
return context.isSingleton(name);
}
public static Class> getType(String name) throws NoSuchBeanDefinitionException {
return context.getType(name);
}
public static String[] getAliases(String name) throws NoSuchBeanDefinitionException {
return context.getAliases(name);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy