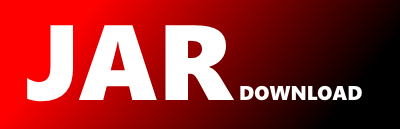
com.feingto.cloud.kit.FileKit Maven / Gradle / Ivy
package com.feingto.cloud.kit;
import lombok.SneakyThrows;
import org.apache.commons.collections.CollectionUtils;
import org.apache.commons.lang3.ArrayUtils;
import org.springframework.util.Assert;
import java.io.*;
import java.util.ArrayList;
import java.util.List;
import java.util.Objects;
import java.util.zip.ZipEntry;
import java.util.zip.ZipOutputStream;
/**
* 文件操作工具类
*
* @author longfei
*/
public class FileKit {
/**
* 获取文件后缀名
*
* @param fileName 文件名
* @return 文件后缀名
*/
public static String getFileExt(String fileName) {
Assert.hasText(fileName, "文件不存在");
int lastIndex = fileName.lastIndexOf(".");
return lastIndex >= 0 ? fileName.substring(lastIndex + 1).toLowerCase() : "";
}
/**
* 获取不包含后缀的文件名字
*
* @param fileName 文件名
* @return String
*/
public static String getRealName(String fileName) {
Assert.hasText(fileName, "文件名不能为空");
int endIndex = fileName.lastIndexOf(".");
return endIndex == -1 ? "" : fileName.substring(0, endIndex);
}
/**
* 文件流上传
*
* @param input InputStream
* @param path 上传路径
*/
@SneakyThrows
public static void uploadFile(InputStream input, String path) {
OutputStream os;
os = new FileOutputStream(new File(path));
int len;
byte[] b = new byte[1024];
while ((len = input.read(b)) != -1) {
os.write(b, 0, len);
}
os.flush();
os.close();
}
/**
* 复制文件流
*
* @param input 输入流
*/
@SneakyThrows
public static ByteArrayInputStream copy(InputStream input) {
ByteArrayOutputStream baos = new ByteArrayOutputStream();
byte[] buffer = new byte[1024];
int len;
while ((len = input.read(buffer)) > -1) {
baos.write(buffer, 0, len);
}
baos.flush();
return new ByteArrayInputStream(baos.toByteArray());
}
/**
* 剪切文件到目标路径
*
* @param source 源路径
* @param target 目标路径
*/
@SneakyThrows
public static void cutFile(String source, String target) {
Assert.hasText(source, "源路径不能为空");
Assert.hasText(target, "目标路径不能为空");
String fileName = source.substring(source.lastIndexOf("/") + 1);
String srcPath = source.substring(0, source.lastIndexOf("/"));
if (srcPath.equals(target)) {
return;
}
File srcFile = new File(srcPath.replace("/", File.separator), fileName);
File destFile = new File(target.replace("/", File.separator), fileName);
if (srcFile.exists()) {
org.apache.commons.io.FileUtils.moveFile(srcFile, destFile);
}
}
/**
* 删除文件
*
* @param path 文件路径
*/
@SneakyThrows
public static void deleteFile(String path) {
Assert.hasText(path, "目录不存在");
File file = new File(path);
if (file.exists()) {
org.apache.commons.io.FileUtils.forceDelete(file);
}
}
/**
* 删除文件夹fileFolder
*
* @param path 文件路径
* @param exclude 排除文件名
*/
@SneakyThrows
public static void deleteFiles(String path, List exclude) {
File directory = new File(path);
if (!directory.exists() || !directory.isDirectory()) {
return;
}
String[] names = directory.list();
if (ArrayUtils.isNotEmpty(names)) {
File file;
for (String name : names) {
if (CollectionUtils.isEmpty(exclude) || !exclude.contains(name)) {
String subName = path + File.separator + name;
file = new File(subName);
if (file.isFile()) {
org.apache.commons.io.FileUtils.forceDelete(file);
} else {
deleteFiles(subName, exclude);
}
}
}
}
}
/**
* 统计路径下的文件数量(不递归)
*
* @param path 文件路径
* @return Integer
*/
public static Integer countFiles(String path) {
int num = 0;
File directory = new File(path);
if (directory.exists() && directory.isDirectory()) {
String[] names = directory.list();
num = Objects.nonNull(names) ? names.length : 0;
}
return num;
}
/**
* 获取路径下的全部文件
*
* @param path 文件路径
* @return 文件集合
*/
public static List getFiles(String path) {
List fileList = new ArrayList<>();
File directory = new File(path);
if (directory.exists() && directory.isDirectory()) {
File[] files = directory.listFiles();
if (ArrayUtils.isNotEmpty(files)) {
for (File file : files) {
if (file.isDirectory()) {
fileList.addAll(getFiles(file.getPath()));
} else {
fileList.add(file);
}
}
}
}
return fileList;
}
/**
* ZIP压缩源文件夹及其全部子文件
*
* @param source 源文件夹路径
*/
@SneakyThrows
public static void ZIP(String source) {
FileOutputStream fos = new FileOutputStream(new File(source + ".zip"));
ZipOutputStream zos = new ZipOutputStream(fos);
File file = new File(source);
if (file.isDirectory()) {
ZIPDir(source, file.getName(), zos);
}
zos.close();
fos.close();
}
/**
* 将目录和该目录下的文件写入ZipOutputStream
*
* @param source 源文件夹
* @param dir 目标文件夹
* @param zos ZipOutputStream
*/
@SneakyThrows
public static void ZIPDir(String source, String dir, ZipOutputStream zos) {
File[] files = new File(source).listFiles();
if (Objects.nonNull(files)) {
zos.putNextEntry(new ZipEntry(dir + File.separator));
for (File subFile : files) {
if (subFile.isDirectory()) {
ZIPDir(subFile.getPath(), dir + File.separator + subFile.getName(), zos);
} else {
ZIPFile(subFile.getPath(), dir + File.separator + subFile.getName(), zos);
}
}
}
}
/**
* 将文件写入ZipOutputStream
*
* @param source 源文件
* @param target 目标文件
* @param zos ZipOutputStream
*/
@SneakyThrows
public static void ZIPFile(String source, String target, ZipOutputStream zos) {
zos.putNextEntry(new ZipEntry(target));
FileInputStream fis = new FileInputStream(new File(source));
byte[] bf = new byte[2048];
int position;
while ((position = fis.read(bf)) != -1) {
zos.write(bf, 0, position);
}
fis.close();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy