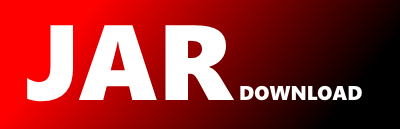
com.feingto.cloud.kit.ObjectKit Maven / Gradle / Ivy
package com.feingto.cloud.kit;
import com.feingto.cloud.kit.json.JSON;
import com.feingto.cloud.kit.reflection.ReflectionKit;
import org.apache.commons.beanutils.BeanUtils;
import java.io.*;
import java.lang.reflect.AccessibleObject;
import java.lang.reflect.Array;
import java.lang.reflect.Field;
import java.lang.reflect.InvocationTargetException;
import java.math.BigDecimal;
import java.util.*;
/**
* 对象转换工具
*
* @author longfei
*/
@SuppressWarnings("unchecked")
public class ObjectKit {
private static final String[] IGNORE_FIELD_NAMES = new String[]{"log", "logger", "serialVersionUID"};
private static final String[] IGNORE_FIELD_CLASSES = new String[]{"org.apache.log4j.Logger", "org.slf4j.Logger",
"org.slf4j.LoggerFactory", "java.kit.logging.Logger", "java.lang.Object", "java.lang.Class",
"java.lang.reflect.AccessibleObject", "java.lang.reflect.Field", "java.lang.reflect.Method",
"java.lang.reflect.Constructor"};
/**
* Returns a copy of the object, or null if the object cannot be serialized.
*
* @param obj Object
* @return a deep copy of that Object
* @throws NotSerializableException if an error occurs
*/
public static Object copy(Object obj) throws NotSerializableException {
Object _obj = null;
try {
ByteArrayOutputStream bos = new ByteArrayOutputStream();
ObjectOutputStream out = new ObjectOutputStream(bos);
out.writeObject(obj);
out.flush();
out.close();
ObjectInputStream in = new ObjectInputStream(new ByteArrayInputStream(bos.toByteArray()));
_obj = in.readObject();
} catch (NotSerializableException e) {
throw e;
} catch (IOException | ClassNotFoundException e) {
e.printStackTrace();
}
return _obj;
}
/**
* 深复制
*
* @param bean Object
* @return T
*/
public static T clone(Object bean) {
return clone(bean, (Class) bean.getClass());
}
/**
* 深复制
*
* @param bean Collection
* @return List
*/
public static List clone(Collection bean) {
return clone(bean, (Class) bean.getClass());
}
/**
* 深复制
*
* @param bean Object
* @param clazz Class
* @return T
*/
public static T clone(Object bean, Class clazz) {
return JSON.json2pojo(JSON.obj2json(bean), clazz);
}
/**
* 深复制
*
* @param bean Collection
* @param clazz Class
* @return List
*/
public static List clone(Collection bean, Class clazz) {
return JSON.json2list(JSON.obj2json(bean), clazz);
}
/**
* 判断对象是否为空
*
* @param obj Object
* @return boolean
*/
public static boolean isEmpty(Object obj) {
return Objects.isNull(obj) || obj.getClass().isArray() && Array.getLength(obj) == 0 ||
obj instanceof Collection> && ((Collection>) obj).isEmpty() ||
obj instanceof Map, ?> && ((Map, ?>) obj).isEmpty();
}
/**
* 对象转换集合
*
* @param obj Object
* @return boolean
*/
public static Collection objectToCollection(Object obj) {
if (Objects.isNull(obj) || obj == "") {
return new ArrayList();
}
Collection collection;
if (obj instanceof Collection) {
collection = (Collection) obj;
} else if (obj instanceof Map) {
collection = ((Map) obj).entrySet();
} else if (obj.getClass().isArray()) {
collection = new ArrayList();
int length = Array.getLength(obj);
for (int i = 0; i < length; i++) {
Object value = Array.get(obj, i);
collection.add(value);
}
} else {
collection = new ArrayList();
collection.add(obj);
}
return collection;
}
public static Object[] collectionToObject(Collection collection) {
return collection.toArray(new Object[0]);
}
public static T mapToObject(Class clazz, Map map) {
try {
T m = clazz.newInstance();
BeanUtils.populate(m, map);
return m;
} catch (IllegalAccessException | InvocationTargetException | InstantiationException e) {
e.printStackTrace();
return null;
}
}
public static List mapToList(Class clazz, List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy