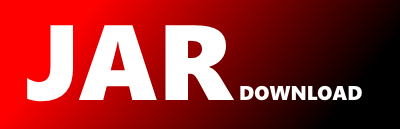
com.feingto.iot.client.handler.MqttClientChannelHandler Maven / Gradle / Ivy
package com.feingto.iot.client.handler;
import com.feingto.iot.client.MqttClient;
import com.feingto.iot.common.handler.DefaultSimpleChannelHandler;
import com.feingto.iot.common.model.mqtt.MqttConnectOptions;
import io.netty.channel.ChannelHandler.Sharable;
import io.netty.channel.ChannelHandlerContext;
import io.netty.handler.codec.mqtt.MqttConnAckMessage;
import io.netty.handler.codec.mqtt.MqttMessage;
import io.netty.handler.codec.mqtt.MqttSubAckMessage;
import io.netty.handler.timeout.IdleState;
import io.netty.handler.timeout.IdleStateEvent;
import java.util.Optional;
import java.util.concurrent.TimeUnit;
/**
* MQTT 客户端消息通道处理器
*
* @author longfei
*/
@Sharable
public class MqttClientChannelHandler extends DefaultSimpleChannelHandler {
private final MqttConnectOptions options;
private final MqttClient mqttClient;
public MqttClientChannelHandler(MqttConnectOptions options, MqttClient mqttClient) {
this.options = options;
this.mqttClient = mqttClient;
}
@Override
public void channelActive(ChannelHandlerContext ctx) {
mqttClient.connect(ctx.channel(), options);
}
@Override
public void channelInactive(ChannelHandlerContext ctx) throws Exception {
ctx.channel().eventLoop()
.schedule(mqttClient::reconnect, options.getRetryInterval(), TimeUnit.SECONDS);
super.channelInactive(ctx);
}
@Override
public void handleMessage(ChannelHandlerContext ctx, MqttMessage msg) {
Optional.ofNullable(msg.fixedHeader()).ifPresent(fixedHeader -> {
switch (fixedHeader.messageType()) {
case CONNACK:
mqttClient.connack((MqttConnAckMessage) msg);
break;
case PUBLISH:
mqttClient.publish(ctx.channel(), msg);
break;
case PUBACK:
mqttClient.puback(ctx.channel(), msg);
break;
case PUBREC:
mqttClient.pubrec(ctx.channel(), msg);
break;
case PUBREL:
mqttClient.pubrel(ctx.channel(), msg);
break;
case PUBCOMP:
mqttClient.pubcomp(ctx.channel(), msg);
break;
case SUBACK:
mqttClient.suback(ctx.channel(), (MqttSubAckMessage) msg);
break;
case UNSUBACK:
mqttClient.unsubBack(ctx.channel(), msg);
break;
default:
break;
}
});
}
@Override
public void userEventTriggered(ChannelHandlerContext ctx, Object evt) throws Exception {
if (evt instanceof IdleStateEvent) {
IdleStateEvent event = (IdleStateEvent) evt;
if (event.state() == IdleState.WRITER_IDLE) {
mqttClient.pingreq(ctx.channel());
}
} else {
super.userEventTriggered(ctx, evt);
}
}
@Override
public void exceptionCaught(ChannelHandlerContext ctx, Throwable cause) {
ctx.channel().eventLoop()
.schedule(mqttClient::reconnect, options.getRetryInterval(), TimeUnit.SECONDS);
mqttClient.exceptionCaught(cause);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy