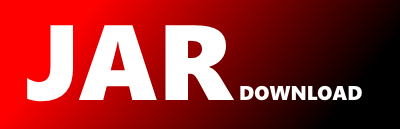
com.feingto.iot.client.bootstrap.BaseBootstrap Maven / Gradle / Ivy
package com.feingto.iot.client.bootstrap;
import com.feingto.iot.client.MqttClient;
import com.feingto.iot.client.config.NettyProperties;
import com.feingto.iot.client.handler.MqttConnectListener;
import com.feingto.iot.common.model.custom.LoginMessage;
import com.feingto.iot.common.model.enums.TermType;
import com.feingto.iot.common.model.mqtt.MqttConnectOptions;
import io.netty.bootstrap.Bootstrap;
import io.netty.channel.Channel;
import io.netty.channel.ChannelFuture;
import io.netty.channel.EventLoopGroup;
import lombok.Setter;
import lombok.extern.slf4j.Slf4j;
import org.apache.commons.codec.digest.DigestUtils;
import java.util.Random;
/**
* 启动器
*
* @author longfei
*/
@Slf4j
public abstract class BaseBootstrap {
protected final NettyProperties config;
protected EventLoopGroup group;
protected MqttClient mqttClient;
@Setter
private boolean initMessage = false;
public BaseBootstrap(NettyProperties config) {
this.config = config;
}
private void sendMessage(Channel channel) {
int id = new Random().nextInt(100);
channel.writeAndFlush(new LoginMessage()
.id(Integer.toString(id))
.token("token-" + id)
.username("guest-" + id)
.password(DigestUtils.md5Hex("123456"))
.type(TermType.IOS.getValue()));
}
public abstract Bootstrap init();
public Channel start() {
MqttConnectOptions options = config.getMqtt();
// Bind and start to connect to client
ChannelFuture future;
try {
future = init()
.connect(options.getHost(), options.getPort())
.addListener(new MqttConnectListener(options, mqttClient))
.sync();
if (initMessage && future.channel().isActive()) {
sendMessage(future.channel());
}
log.info("IoT client listening on port [{}]", options.getPort());
return future.channel();
} catch (InterruptedException e) {
log.error("IoT client exception: {}", e.getMessage());
throw new RuntimeException(e.getCause());
}
}
public void close() {
log.info("IoT client shutdown");
group.shutdownGracefully();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy