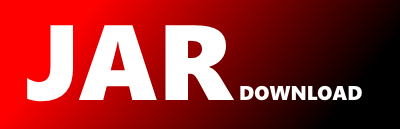
com.feingto.iot.client.bootstrap.ClientBootstrap Maven / Gradle / Ivy
package com.feingto.iot.client.bootstrap;
import com.feingto.iot.client.handler.ClientMessageHandler;
import com.feingto.iot.common.bootstrap.SimpleHandlerLoader;
import com.feingto.iot.common.config.properties.NettyProperties;
import com.feingto.iot.common.model.custom.LoginMessage;
import com.feingto.iot.common.model.enums.TermType;
import io.netty.bootstrap.Bootstrap;
import io.netty.channel.*;
import io.netty.channel.nio.NioEventLoopGroup;
import io.netty.channel.socket.SocketChannel;
import io.netty.channel.socket.nio.NioSocketChannel;
import io.netty.handler.timeout.IdleStateHandler;
import io.netty.util.concurrent.DefaultThreadFactory;
import lombok.extern.slf4j.Slf4j;
import org.apache.commons.codec.digest.DigestUtils;
import org.springframework.stereotype.Component;
import javax.annotation.Resource;
import java.util.List;
import java.util.Random;
import java.util.concurrent.TimeUnit;
/**
* IoT 客户端启动器
*
* @author longfei
*/
@Slf4j
@Component
public class ClientBootstrap {
@Resource
private NettyProperties config;
public void connect() {
EventLoopGroup group = new NioEventLoopGroup(config.getWorkThread(), new DefaultThreadFactory("CLIENT_"));
try {
Bootstrap bootstrap = new Bootstrap();
bootstrap.group(group)
.channel(NioSocketChannel.class)
.option(ChannelOption.TCP_NODELAY, true)
.handler(new ChannelInitializer() {
@Override
protected void initChannel(SocketChannel ch) {
List handlers = SimpleHandlerLoader.getTcpHandlers();
handlers.add(new IdleStateHandler(0, 1, 0, TimeUnit.MINUTES));
handlers.add(new ClientMessageHandler());
handlers.forEach(handler -> ch.pipeline().addLast(handler));
}
});
// Bind and start to connect to client
ChannelFuture future = bootstrap.connect(config.getHost(), config.getPort()).sync();
log.info("IoT client listening on port [{}]", config.getPort());
future.awaitUninterruptibly(2000, TimeUnit.MILLISECONDS);
if (future.channel().isActive()) {
int id = new Random().nextInt(100);
future.channel().writeAndFlush(new LoginMessage()
.id(Integer.toString(id))
.token("token-" + id)
.username("guest-" + id)
.password(DigestUtils.md5Hex("123456"))
.type(TermType.IOS.getValue()));
}
} catch (InterruptedException e) {
e.printStackTrace();
log.error("IoT client exception: {}", e.getMessage());
} finally {
log.info("IoT client closing...");
group.shutdownGracefully();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy