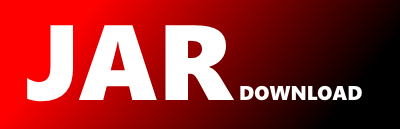
com.feingto.iot.common.bootstrap.MqttConsumerBootstrap Maven / Gradle / Ivy
package com.feingto.iot.common.bootstrap;
import com.feingto.iot.common.config.properties.NettyProperties;
import com.feingto.iot.common.handler.MessageConsumeChannelHandler;
import com.feingto.iot.common.service.mqtt.IMessageConsume;
import io.netty.bootstrap.Bootstrap;
import io.netty.channel.*;
import io.netty.channel.nio.NioEventLoopGroup;
import io.netty.channel.socket.SocketChannel;
import io.netty.channel.socket.nio.NioSocketChannel;
import io.netty.handler.timeout.IdleStateHandler;
import io.netty.util.concurrent.DefaultThreadFactory;
import lombok.Setter;
import lombok.experimental.Accessors;
import lombok.extern.slf4j.Slf4j;
import javax.annotation.PreDestroy;
import java.util.concurrent.TimeUnit;
/**
* MQTT 消费者启动器
*
* @author longfei
*/
@Slf4j
@Accessors(fluent = true)
public class MqttConsumerBootstrap {
private final NettyProperties config;
private EventLoopGroup group;
@Setter
private IMessageConsume messageService;
public MqttConsumerBootstrap(NettyProperties config) {
this.config = config;
}
public Channel start() throws InterruptedException {
group = new NioEventLoopGroup(config.getWorkThread(), new DefaultThreadFactory("CLIENT_"));
NettyProperties.Mqtt mqtt = config.getMqtt();
Bootstrap bootstrap = new Bootstrap()
.group(group)
.channel(NioSocketChannel.class)
.option(ChannelOption.TCP_NODELAY, true)
.option(ChannelOption.CONNECT_TIMEOUT_MILLIS, mqtt.getTimeout())
.handler(new ChannelInitializer() {
@Override
protected void initChannel(SocketChannel ch) {
if (mqtt.getKeepAliveInterval() > 0) {
ch.pipeline()
.addLast(new IdleStateHandler(0,
mqtt.getKeepAliveInterval(), 0, TimeUnit.SECONDS));
}
SimpleHandlerLoader.getMqttChannelHandlers()
.forEach(ch.pipeline()::addLast);
if (messageService != null) {
ch.pipeline().addLast(new MessageConsumeChannelHandler(mqtt, messageService));
}
}
});
// Bind and start to connect to client
ChannelFuture future = bootstrap.connect(mqtt.getHost(), mqtt.getPort()).sync();
if (future.isDone()) {
log.info("IoT client listening on port [{}]", mqtt.getPort());
}
return future.channel();
}
@PreDestroy
public void close() {
log.info("IoT client closing...");
group.shutdownGracefully();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy