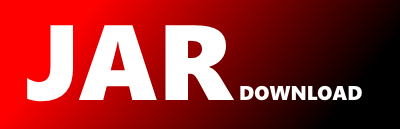
com.feingto.iot.common.handler.DefaultChannelInboundHandler Maven / Gradle / Ivy
package com.feingto.iot.common.handler;
import com.feingto.iot.common.Constants;
import com.feingto.iot.common.cache.ChannelCache;
import io.netty.channel.ChannelHandlerContext;
import io.netty.channel.ChannelInboundHandlerAdapter;
import io.netty.handler.codec.mqtt.MqttMessage;
import lombok.extern.slf4j.Slf4j;
/**
* 通道Inbound处理器抽象
*
* @author longfei
*/
@Slf4j
public abstract class DefaultChannelInboundHandler extends ChannelInboundHandlerAdapter {
public abstract void handleMessage(ChannelHandlerContext ctx, Object msg);
/**
* 当一个Channel注册到EventLoop上,可以处理I/O时被调用
*/
@Override
public void channelRegistered(ChannelHandlerContext ctx) {
log.info("{} registered, current connected: {}", ctx.channel().remoteAddress(),
Constants.CONNECT_COUNTER.incrementAndGet());
}
/**
* 当一个Channel从它的EventLoop上解除注册,不再处理I/O时被调用
*/
@Override
public void channelUnregistered(ChannelHandlerContext ctx) {
log.info("{} unregistered, current connected: {}", ctx.channel().remoteAddress(),
Constants.CONNECT_COUNTER.decrementAndGet());
}
/**
* 当Channel离开活跃状态,不再连接到某个远端时被调用
*/
@Override
public void channelInactive(ChannelHandlerContext ctx) {
ChannelCache.getInstance().remove(ctx.channel().id().asLongText());
}
/**
* 当从Channel中读数据时被调用
*/
@Override
public void channelRead(ChannelHandlerContext ctx, Object msg) {
if (msg instanceof MqttMessage) {
ctx.fireChannelRead(msg);
} else {
handleMessage(ctx, msg);
}
}
/**
* 当Channel上的某个读操作完成时被调用
*/
@Override
public void channelReadComplete(ChannelHandlerContext ctx) {
ctx.flush();
}
/**
* 处理过程中ChannelPipeline中发生错误时被调用
*/
@Override
public void exceptionCaught(ChannelHandlerContext ctx, Throwable cause) throws Exception {
super.exceptionCaught(ctx, cause);
cause.printStackTrace();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy