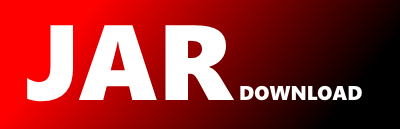
com.feingto.iot.common.handler.MessageConsumeChannelHandler Maven / Gradle / Ivy
package com.feingto.iot.common.handler;
import com.feingto.iot.common.config.properties.NettyProperties;
import com.feingto.iot.common.service.mqtt.IMessageConsume;
import io.netty.channel.ChannelHandler.Sharable;
import io.netty.channel.ChannelHandlerContext;
import io.netty.channel.SimpleChannelInboundHandler;
import io.netty.handler.codec.mqtt.*;
import io.netty.handler.timeout.IdleState;
import io.netty.handler.timeout.IdleStateEvent;
import java.util.Optional;
/**
* 消息消费通道处理器
*
* @author longfei
*/
@Sharable
public class MessageConsumeChannelHandler extends SimpleChannelInboundHandler {
private final NettyProperties.Mqtt mqtt;
private final IMessageConsume clientService;
public MessageConsumeChannelHandler(NettyProperties.Mqtt mqtt, IMessageConsume clientService) {
this.mqtt = mqtt;
this.clientService = clientService;
}
@Override
protected void channelRead0(ChannelHandlerContext ctx, MqttMessage msg) {
Optional.ofNullable(msg.fixedHeader()).ifPresent(fixedHeader -> {
switch (fixedHeader.messageType()) {
case CONNACK:
clientService.connack((MqttConnAckMessage) msg);
break;
case PUBLISH:
clientService.publish(ctx.channel(), msg);
break;
case PUBACK:
clientService.puback(ctx.channel(), msg);
break;
case PUBREC:
clientService.pubrec(ctx.channel(), msg);
break;
case PUBREL:
clientService.pubrel(ctx.channel(), msg);
break;
case PUBCOMP:
clientService.pubcomp(ctx.channel(), msg);
break;
case SUBACK:
clientService.suback(ctx.channel(), (MqttSubAckMessage) msg);
break;
case UNSUBACK:
clientService.unsubBack(ctx.channel(), msg);
break;
default:
break;
}
});
}
@Override
public void channelActive(ChannelHandlerContext ctx) {
ctx.channel().writeAndFlush(new MqttConnectMessage(
new MqttFixedHeader(MqttMessageType.CONNECT, false,
MqttQoS.AT_LEAST_ONCE, false, 10),
new MqttConnectVariableHeader(
MqttVersion.MQTT_3_1_1.protocolName(), MqttVersion.MQTT_3_1_1.protocolLevel(),
true, true,
mqtt.isWillRetain(), mqtt.getWillQos(), mqtt.isWillFlag(),
mqtt.isCleanSession(), mqtt.getKeepAliveInterval()),
new MqttConnectPayload(mqtt.getClientId(), mqtt.getWillTopic(),
mqtt.getWillMessage().getBytes(), mqtt.getUsername(), mqtt.getPassword().getBytes())));
}
@Override
public void channelInactive(ChannelHandlerContext ctx) {
clientService.disconnect(ctx.channel());
ctx.flush();
}
@Override
public void userEventTriggered(ChannelHandlerContext ctx, Object evt) throws Exception {
if (evt instanceof IdleStateEvent) {
IdleStateEvent event = (IdleStateEvent) evt;
if (event.state() == IdleState.WRITER_IDLE) {
clientService.pingreq(ctx.channel());
}
} else {
super.userEventTriggered(ctx, evt);
}
}
@Override
public void exceptionCaught(ChannelHandlerContext ctx, Throwable cause) throws Exception {
super.exceptionCaught(ctx, cause);
clientService.exceptionCaught(cause);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy