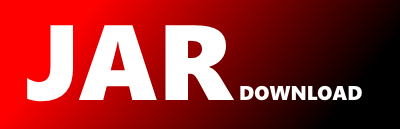
com.feingto.iot.common.service.mqtt.MessageRequest Maven / Gradle / Ivy
package com.feingto.iot.common.service.mqtt;
import com.feingto.iot.common.model.mqtt.SendMessage;
import io.netty.buffer.Unpooled;
import io.netty.channel.Channel;
import io.netty.handler.codec.mqtt.*;
import lombok.extern.slf4j.Slf4j;
import java.util.List;
/**
* 消息请求
*
* @author longfei
*/
@Slf4j
public class MessageRequest {
/**
* 推送消息
*/
public static void publish(Channel channel, SendMessage msg) {
log.debug(">>> publish to topic: {} of message: {}", msg.topic(), new String(msg.payload()));
channel.writeAndFlush(new MqttPublishMessage(
new MqttFixedHeader(MqttMessageType.PUBLISH, msg.dup(),
msg.mqttQoS(), msg.retain(), 0),
new MqttPublishVariableHeader(msg.topic(), msg.id()),
Unpooled.wrappedBuffer(msg.payload())));
}
/**
* 订阅消息
*/
public static void subscribe(Channel channel, List mqttTopicSubscriptions, int messageId) {
channel.writeAndFlush(new MqttSubscribeMessage(
new MqttFixedHeader(MqttMessageType.SUBSCRIBE, false,
MqttQoS.AT_LEAST_ONCE, false, 0),
MqttMessageIdVariableHeader.from(messageId),
new MqttSubscribePayload(mqttTopicSubscriptions)));
}
/**
* 取消订阅消息
*/
public static void unsubscribe(Channel channel, List topic, int messageId) {
channel.writeAndFlush(new MqttUnsubscribeMessage(
new MqttFixedHeader(MqttMessageType.UNSUBSCRIBE, false,
MqttQoS.AT_LEAST_ONCE, false, 0x02),
MqttMessageIdVariableHeader.from(messageId),
new MqttUnsubscribePayload(topic)));
}
/**
* PING请求
*/
public static void pingreq(Channel channel) {
channel.writeAndFlush(new MqttMessage(
new MqttFixedHeader(MqttMessageType.PINGREQ, false,
MqttQoS.AT_MOST_ONCE, false, 0)));
}
/**
* 断开连接消息
*/
public static void disconnect(Channel channel) {
channel.writeAndFlush(new MqttMessage(
new MqttFixedHeader(MqttMessageType.DISCONNECT, false,
MqttQoS.AT_LEAST_ONCE, false, 0x02)));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy