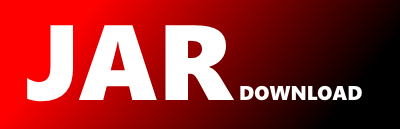
com.feingto.iot.common.bootstrap.SimpleHandlerLoader Maven / Gradle / Ivy
package com.feingto.iot.common.bootstrap;
import com.feingto.iot.common.Constants;
import com.feingto.iot.common.codec.MessageDecoder;
import com.feingto.iot.common.codec.MessageEncoder;
import com.feingto.iot.common.codec.MqttWebSocketCodec;
import io.netty.buffer.Unpooled;
import io.netty.channel.ChannelHandler;
import io.netty.handler.codec.DelimiterBasedFrameDecoder;
import io.netty.handler.codec.LineBasedFrameDecoder;
import io.netty.handler.codec.http.HttpContentCompressor;
import io.netty.handler.codec.http.HttpObjectAggregator;
import io.netty.handler.codec.http.HttpServerCodec;
import io.netty.handler.codec.http.websocketx.WebSocketServerProtocolHandler;
import io.netty.handler.codec.mqtt.MqttDecoder;
import io.netty.handler.codec.mqtt.MqttEncoder;
import io.netty.handler.codec.string.StringDecoder;
import io.netty.handler.codec.string.StringEncoder;
import io.netty.handler.stream.ChunkedWriteHandler;
import io.netty.util.CharsetUtil;
import java.util.ArrayList;
import java.util.List;
/**
* 消息处理加载器
*
* @author longfei
*/
public class SimpleHandlerLoader {
/**
* 回车换行解码处理器,以回车换行符作为消息结束的标识
*/
public static List getLineBasedHandlers() {
List handlers = new ArrayList<>();
handlers.add(new LineBasedFrameDecoder(Integer.MAX_VALUE));
handlers.add(new StringDecoder(CharsetUtil.UTF_8));
handlers.add(new StringEncoder(CharsetUtil.UTF_8));
return handlers;
}
/**
* 分隔符解码处理器,指定消息结束的分隔符,自动完成以分隔符作为码流结束标识的消息的解码
* 回车换行解码处理器实际上是一种特殊的DelimiterBasedFrameDecoder解码器
*/
public static List getDelimiterBasedHandlers() {
List handlers = new ArrayList<>();
handlers.add(new DelimiterBasedFrameDecoder(Integer.MAX_VALUE,
Unpooled.copiedBuffer(Constants.DELIMITER.getBytes())));
handlers.add(new StringDecoder(CharsetUtil.UTF_8));
handlers.add(new StringEncoder(CharsetUtil.UTF_8));
return handlers;
}
/**
* TCP 自定义长度解码处理器
*/
public static List getTcpHandlers() {
List handlers = new ArrayList<>();
handlers.add(new MessageDecoder(Integer.MAX_VALUE, 2, 4,
1, 0, true));
handlers.add(new MessageEncoder());
return handlers;
}
/**
* Http 消息处理器
*/
public static List getHttpChannelHandlers() {
List handlers = new ArrayList<>();
handlers.add(new HttpServerCodec());// Http消息编码解码
handlers.add(new HttpObjectAggregator(65536));// Http消息组装,负责把多个HttpMessage组装成一个完整的Http请求或者响应
handlers.add(new ChunkedWriteHandler());// 处理大文件传输
return handlers;
}
/**
* MQTT 消息处理器
*/
public static List getMqttChannelHandlers() {
List handlers = new ArrayList<>();
handlers.add(new MqttDecoder());
handlers.add(MqttEncoder.INSTANCE);
return handlers;
}
/**
* MQTT Websocket 消息处理器
*/
public static List getMqttWsChannelHandlers() {
List handlers = new ArrayList<>();
handlers.add(new HttpServerCodec());
handlers.add(new HttpObjectAggregator(65536));
handlers.add(new HttpContentCompressor());
handlers.add(new WebSocketServerProtocolHandler("/mqtt", "mqtt, mqttv3.1, mqttv3.1.1"));
handlers.add(new MqttWebSocketCodec());
handlers.addAll(getMqttChannelHandlers());
return handlers;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy