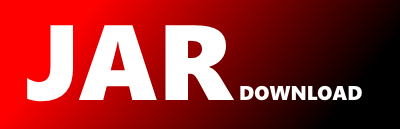
com.feingto.iot.server.bootstrap.IoTServerBootstrap Maven / Gradle / Ivy
package com.feingto.iot.server.bootstrap;
import com.feingto.iot.common.bootstrap.SimpleHandlerLoader;
import com.feingto.iot.common.model.enums.Protocol;
import com.feingto.iot.server.config.properties.NettyProperties;
import com.feingto.iot.server.handler.HttpRequestHandler;
import com.feingto.iot.server.handler.MqttMessageHandler;
import com.feingto.iot.server.handler.TcpMessageHandler;
import com.feingto.iot.server.handler.WebSocketMessageHandler;
import com.feingto.iot.server.util.SslContextLoader;
import io.netty.bootstrap.ServerBootstrap;
import io.netty.channel.*;
import io.netty.channel.nio.NioEventLoopGroup;
import io.netty.channel.socket.SocketChannel;
import io.netty.channel.socket.nio.NioServerSocketChannel;
import io.netty.handler.logging.LogLevel;
import io.netty.handler.logging.LoggingHandler;
import io.netty.handler.ssl.SslHandler;
import io.netty.handler.timeout.IdleStateHandler;
import io.netty.util.concurrent.DefaultThreadFactory;
import lombok.Setter;
import lombok.experimental.Accessors;
import lombok.extern.slf4j.Slf4j;
import javax.net.ssl.SSLEngine;
import java.util.concurrent.TimeUnit;
/**
* IoT 服务启动器
*
* @author longfei
*/
@Slf4j
@Accessors(fluent = true)
public class IoTServerBootstrap {
@Setter
private Protocol protocol;
@Setter
private int port;
@Setter
private NettyProperties config;
public void run() {
EventLoopGroup bossGroup = new NioEventLoopGroup(config.getBossThread(), new DefaultThreadFactory("BOSS_"));
EventLoopGroup workGroup = new NioEventLoopGroup(config.getWorkThread(), new DefaultThreadFactory("WORK_"));
try {
ServerBootstrap bootstrap = new ServerBootstrap();
bootstrap.group(bossGroup, workGroup)
.channel(NioServerSocketChannel.class)
.option(ChannelOption.SO_BACKLOG, config.getBacklog())
.childOption(ChannelOption.SO_KEEPALIVE, config.isKeepalive())
.handler(new LoggingHandler(LogLevel.INFO))
.childHandler(new ChannelInitializer() {
@Override
protected void initChannel(SocketChannel ch) {
if (config.isSsl()) {
SSLEngine engine = SslContextLoader
.initialize(config.getKeyStore(), config.getKeyStorePassword())
.newEngine(ch.alloc());
engine.setUseClientMode(false);
engine.setNeedClientAuth(false);
ch.pipeline().addLast(new SslHandler(engine));
}
intProtocolHandler(ch.pipeline());
}
});
// Bind and start to accept incoming connections
Channel channel = bootstrap.bind(port).sync().channel();
switch (this.protocol) {
case TCP:
log.info("[{}] Accepting connections at: {}", protocol, "tcp://0.0.0.0:" + port);
break;
case WS:
log.info("[{}] Accepting connections at: {}", protocol, "ws://0.0.0.0:" + port + "/websocket");
break;
case MQTT:
log.info("[{}] Accepting connections at: {}", protocol, "tcp://0.0.0.0:" + port);
break;
case MQTT_WS:
log.info("[{}] Accepting connections at: {}", protocol, "ws://0.0.0.0:" + port + "/mqtt");
break;
}
// Wait until the server socket is closed
channel.closeFuture().sync();
} catch (Exception e) {
log.error("IoT [{}] server exception: {}", protocol, e.getMessage());
throw new RuntimeException(e.getCause());
} finally {
log.info("IoT [{}] server closing...", protocol);
bossGroup.shutdownGracefully();
workGroup.shutdownGracefully();
}
}
private void intProtocolHandler(ChannelPipeline channelPipeline) {
switch (this.protocol) {
case TCP:
SimpleHandlerLoader.getTcpHandlers().forEach(channelPipeline::addLast);
channelPipeline.addLast(new IdleStateHandler(config.getMqtt().getTimeout(),
0, 0, TimeUnit.SECONDS));
channelPipeline.addLast(new TcpMessageHandler());
break;
case WS:
SimpleHandlerLoader.getHttpChannelHandlers().forEach(channelPipeline::addLast);
channelPipeline.addLast(new WebSocketMessageHandler());
channelPipeline.addLast(new HttpRequestHandler());
break;
case MQTT:
SimpleHandlerLoader.getMqttChannelHandlers().forEach(channelPipeline::addLast);
channelPipeline.addLast(new MqttMessageHandler());
break;
case MQTT_WS:
SimpleHandlerLoader.getMqttWsChannelHandlers().forEach(channelPipeline::addLast);
channelPipeline.addLast(new MqttMessageHandler());
break;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy