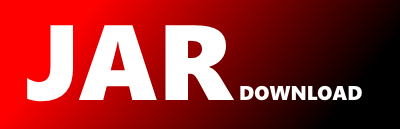
com.feingto.iot.server.cache.ChannelCache Maven / Gradle / Ivy
package com.feingto.iot.server.cache;
import com.feingto.iot.server.serialize.JSON;
import com.feingto.iot.common.model.custom.ChartMessage;
import com.feingto.iot.common.model.custom.Message;
import com.feingto.iot.common.model.custom.MessageChannel;
import com.feingto.iot.common.util.DateKit;
import io.netty.handler.codec.http.websocketx.TextWebSocketFrame;
import org.springframework.util.Assert;
import org.springframework.util.StringUtils;
import java.util.concurrent.ConcurrentHashMap;
/**
* 通道缓存
*
* @author longfei
*/
public class ChannelCache {
private static ChannelCache instance;
private static ConcurrentHashMap channelCache = new ConcurrentHashMap<>();
public static ChannelCache getInstance() {
if (instance == null) {
instance = new ChannelCache();
}
return instance;
}
public MessageChannel get(String key) {
return channelCache.get(key);
}
public void put(String key, MessageChannel message) {
Assert.notNull(key, "The key parameter cannot be empty");
channelCache.put(key, message);
}
public void remove(String key) {
Assert.notNull(key, "The key parameter cannot be empty");
channelCache.remove(key);
}
/**
* 推送消息
*/
public void push(Message message) {
channelCache.values().forEach(mc -> mc.channel().writeAndFlush(message));
}
/**
* 推送聊天消息
*/
public void push(ChartMessage message) {
TextWebSocketFrame text = new TextWebSocketFrame(JSON.getInstance().obj2json(message.timestamp(DateKit.nowUTC())));
if (StringUtils.isEmpty(message.to())) {
// 群发
channelCache.values().stream()
.filter(mc -> !mc.username().equals(message.from()))
.forEach(mc -> mc.channel().writeAndFlush(text));
} else {
// 单发
channelCache.values().stream()
.filter(mc -> mc.username().equals(message.to()))
.forEach(mc -> mc.channel().writeAndFlush(text));
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy