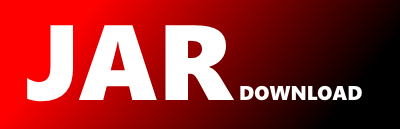
com.feingto.iot.server.cache.RetainedCache Maven / Gradle / Ivy
package com.feingto.iot.server.cache;
import com.feingto.iot.common.model.mqtt.SendMessage;
import com.feingto.iot.common.util.MatchingKit;
import org.apache.ignite.IgniteCache;
import org.springframework.util.Assert;
import java.util.concurrent.atomic.AtomicReference;
/**
* MQTT 保留消息缓存
* 存储每个Topic的最后一条保留消息及其Qos
*
* @author longfei
*/
public class RetainedCache {
private static RetainedCache instance;
/**
* 保留消息Map, key: topic
*/
private static IgniteCache igniteCache;
public static RetainedCache getInstance(IgniteCache cache) {
if (instance == null) {
instance = new RetainedCache();
igniteCache = cache;
}
return instance;
}
/**
* 获取保留消息
*/
public SendMessage get(String topic) {
AtomicReference message = new AtomicReference<>();
igniteCache.forEach(entry -> {
if (MatchingKit.ofTopic(topic, entry.getKey())) {
message.set(igniteCache.get(entry.getKey()));
}
});
return message.get();
}
/**
* 保存保留消息
*/
public void put(String topic, SendMessage message) {
Assert.notNull(topic, "The topic parameter cannot be empty");
if (message.payload().length == 0) {
remove(topic);
} else {
igniteCache.put(topic, message);
}
}
/**
* 移除保留消息
*/
public void remove(String topic) {
Assert.notNull(topic, "The topic parameter cannot be empty");
igniteCache.remove(topic);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy