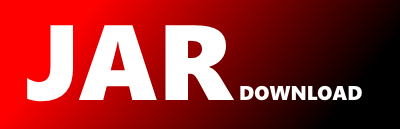
com.feingto.iot.server.cache.SubscribeCache Maven / Gradle / Ivy
package com.feingto.iot.server.cache;
import com.feingto.iot.common.model.mqtt.SubscribeMessage;
import com.feingto.iot.common.util.MatchingKit;
import lombok.extern.slf4j.Slf4j;
import org.apache.ignite.IgniteCache;
import org.springframework.util.Assert;
import java.util.ArrayList;
import java.util.List;
import java.util.concurrent.ConcurrentHashMap;
/**
* Mqtt 订阅主题缓存
*
* @author longfei
*/
@Slf4j
public class SubscribeCache {
private static SubscribeCache instance;
/**
* 订阅主题缓存 [topic, [clientId, SubscribeMessage]]
*/
private static IgniteCache> igniteCache;
public static SubscribeCache getInstance(IgniteCache> cache) {
if (instance == null) {
instance = new SubscribeCache();
igniteCache = cache;
}
return instance;
}
/**
* 根据主题获取订阅
*/
public List findByTopic(String topic) {
List subscribes = new ArrayList<>();
igniteCache.forEach(entry -> {
if (MatchingKit.ofTopic(entry.getKey(), topic)) {
subscribes.addAll(entry.getValue().values());
}
});
log.debug(">>> topic:[{}] match {} subscribes", topic, subscribes.size());
return subscribes;
}
/**
* 根据clientId获取订阅
*/
public List findByClientId(String clientId) {
List subscribes = new ArrayList<>();
igniteCache.forEach(entry -> {
if (entry.getValue().containsKey(clientId)) {
subscribes.add(entry.getValue().get(clientId));
}
});
log.debug(">>> client:[{}] match {} subscribes", clientId, subscribes.size());
return subscribes;
}
/**
* 保存订阅
*/
public void put(String topic, SubscribeMessage message) {
Assert.notNull(topic, "The topic parameter cannot be empty");
ConcurrentHashMap map = igniteCache.containsKey(topic) ?
igniteCache.get(topic) : new ConcurrentHashMap<>();
map.put(message.clientId(), message);
igniteCache.put(topic, map);
}
/**
* 移除clientId订阅
*/
public void remove(String clientId) {
Assert.notNull(clientId, "The clientId parameter cannot be empty");
igniteCache.forEach(entry -> {
ConcurrentHashMap map = entry.getValue();
if (map.containsKey(clientId)) {
map.remove(clientId);
if (map.size() > 0) {
igniteCache.put(entry.getKey(), map);
} else {
igniteCache.remove(entry.getKey());
}
}
});
}
/**
* 移除topic的clientId订阅
*/
public void remove(String topic, String clientId) {
Assert.notNull(topic, "The topic parameter cannot be empty");
Assert.notNull(clientId, "The clientId parameter cannot be empty");
if (igniteCache.containsKey(topic)) {
ConcurrentHashMap map = igniteCache.get(topic);
if (map.containsKey(clientId)) {
map.remove(clientId);
if (map.size() > 0) {
igniteCache.put(topic, map);
} else {
igniteCache.remove(topic);
}
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy