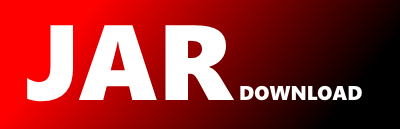
com.feingto.iot.server.codec.RouteChannelDecoder Maven / Gradle / Ivy
package com.feingto.iot.server.codec;
import com.feingto.iot.common.bootstrap.SimpleHandlerLoader;
import com.feingto.iot.server.handler.HttpRequestHandler;
import com.feingto.iot.server.handler.TcpMessageHandler;
import com.feingto.iot.server.handler.WebSocketMessageHandler;
import io.netty.buffer.ByteBuf;
import io.netty.channel.ChannelHandler;
import io.netty.channel.ChannelHandlerContext;
import io.netty.handler.codec.ByteToMessageDecoder;
import io.netty.handler.codec.TooLongFrameException;
import io.netty.handler.codec.http.HttpConstants;
import io.netty.handler.timeout.IdleStateHandler;
import io.netty.util.ByteProcessor;
import io.netty.util.internal.AppendableCharSequence;
import java.util.List;
import java.util.concurrent.TimeUnit;
/**
* 路由通道解码器, 支持单口双工协议 TCP 和 WebSocket
*
* @author longfei
* @see io.netty.handler.codec.http.HttpObjectDecoder
*/
public class RouteChannelDecoder extends ByteToMessageDecoder {
private final LineParser lineParser;
public RouteChannelDecoder() {
this.lineParser = new LineParser(new AppendableCharSequence(128), 4096);
}
@Override
protected void decode(ChannelHandlerContext ctx, ByteBuf in, List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy