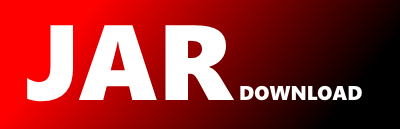
com.feingto.iot.server.handler.TcpMessageHandler Maven / Gradle / Ivy
package com.feingto.iot.server.handler;
import com.feingto.iot.common.handler.DefaultChannelInboundHandler;
import com.feingto.iot.common.model.custom.*;
import com.feingto.iot.common.model.enums.MessageType;
import com.feingto.iot.server.cache.ChannelCache;
import com.google.common.collect.Maps;
import io.netty.channel.ChannelHandler.Sharable;
import io.netty.channel.ChannelHandlerContext;
import io.netty.channel.ChannelId;
import io.netty.handler.timeout.IdleState;
import io.netty.handler.timeout.IdleStateEvent;
import io.netty.util.ReferenceCountUtil;
import lombok.extern.slf4j.Slf4j;
import java.util.Map;
import java.util.concurrent.atomic.AtomicReference;
/**
* TCP 消息处理器
*
* @author longfei
*/
@Slf4j
@Sharable
@SuppressWarnings("unchecked")
public class TcpMessageHandler extends DefaultChannelInboundHandler {
private static AtomicReference
© 2015 - 2025 Weber Informatics LLC | Privacy Policy