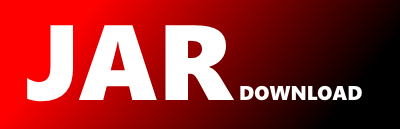
com.feingto.iot.server.util.Push Maven / Gradle / Ivy
package com.feingto.iot.server.util;
import com.feingto.iot.server.serialize.JSON;
import javapns.devices.Device;
import javapns.devices.implementations.basic.BasicDevice;
import javapns.notification.AppleNotificationServerBasicImpl;
import javapns.notification.PushNotificationManager;
import javapns.notification.PushNotificationPayload;
import javapns.notification.PushedNotification;
import lombok.extern.slf4j.Slf4j;
import java.io.InputStream;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
/**
* 推送通知
*
* @author longfei
*/
@Slf4j
public class Push {
/**
* .p12格式的文件路径
*/
private static final String IOS_PATH = "aps_development_cer.p12";
/**
* 证书密码
*/
private static final String IOS_PASSWORD = "toonyoo";
/**
* 是否生产环境
*/
private static final boolean PRODUCTION = false;
/**
* IOS 推送
* 测试推送服务器地址:gateway.sandbox.push.apple.com /2195
* 产品推送服务器地址:gateway.push.apple.com / 2195
*
* @param message 推送内容 {"aps": {"alert": "消息内容"}}
* @param deviceToken 设备token, 用于注册设备到apns, 应用启动时获取到devicetoken并发送到消息服务端
* @return tag 00: 成功; 01: 失败; 02: 部分成功
*/
public String sendpush(String message, String... deviceToken) {
String result;
InputStream path = Thread.currentThread().getContextClassLoader().getResourceAsStream(IOS_PATH);
if (path == null) {
return "01";
}
try {
PushNotificationPayload payLoad = PushNotificationPayload.fromJSON(message);
// 消息内容
String alert = JSON.getInstance().read(message).get("aps").get("alert").asText();
payLoad.addAlert(alert);
// 应用图标上小红圈上的数值
payLoad.addBadge(1);
// 默认铃音
payLoad.addSound("default");
PushNotificationManager pushManager = new PushNotificationManager();
pushManager.initializeConnection(new AppleNotificationServerBasicImpl(path, IOS_PASSWORD, PRODUCTION));
List notifications = new ArrayList<>();
List deviceList = Arrays.asList(deviceToken);
if (deviceList.size() == 1) {
log.debug(">>> push to {}: {}", deviceList.get(0), alert);
PushedNotification notification = pushManager.sendNotification(
new BasicDevice(deviceList.get(0)), payLoad, true);
notifications.add(notification);
} else {
log.debug(">>> push to {}: {}", deviceToken, alert);
List devices = new ArrayList<>();
for (String device : deviceList) {
devices.add(new BasicDevice(device));
}
notifications = pushManager.sendNotifications(payLoad, devices);
}
List failedNotifications = PushedNotification.findFailedNotifications(notifications);
List successfulNotifications = PushedNotification.findSuccessfulNotifications(notifications);
int failed = failedNotifications.size();
int successful = successfulNotifications.size();
if (successful > 0 && failed == 0) {
log.debug(">>> push successful {} times", successfulNotifications.size());
result = "00";
} else if (successful == 0 && failed > 0) {
log.debug(">>> push failed {} times", failedNotifications.size());
result = "01";
} else if (successful == 0) {
log.debug(">>> may be because of a serious error that no notification can be sent");
result = "01";
} else {
log.debug(">>> push successful {} times, failed {} times", successfulNotifications.size(), failedNotifications.size());
result = "02";
}
pushManager.stopConnection();
} catch (Exception e) {
e.printStackTrace();
result = "01";
}
return result;
}
public static void main(String[] args) {
new Push().sendpush("{\"aps\": {\"alert\": \"This is a test message\"}}",
"76edc85fd2e6704b27974d774cc046d7e33a3440fd6f39ba18c729387e6c788a");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy