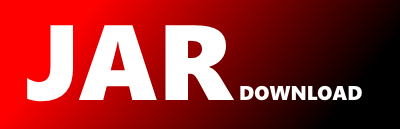
com.feizhaiyou.encrypt.codec.RSAProcessor Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of encrypt-spring-boot-starter Show documentation
Show all versions of encrypt-spring-boot-starter Show documentation
整合SpringBoot可对Web项目的HTTP接口参数进行脱敏与加解密,通过注解的方式直接使用加解密,支持AES、RSA,可自定义脱敏与加解密配置。
The newest version!
package com.feizhaiyou.encrypt.codec;
import cn.hutool.core.codec.Base64Encoder;
import cn.hutool.core.util.CharsetUtil;
import cn.hutool.core.util.HexUtil;
import cn.hutool.core.util.StrUtil;
import cn.hutool.crypto.SecureUtil;
import cn.hutool.crypto.asymmetric.KeyType;
import cn.hutool.crypto.asymmetric.RSA;
import com.feizhaiyou.encrypt.constants.SecurityMode;
import lombok.Data;
import lombok.extern.slf4j.Slf4j;
import org.apache.commons.lang3.StringUtils;
import java.security.KeyPair;
import java.util.HashMap;
import java.util.Map;
/**
* @author ls
*/
@Data
@Slf4j
public class RSAProcessor implements SecurityProcessor {
private String publicKey;
private String privateKey;
private RSA rsa;
public RSAProcessor(String publicKey, String privateKey) {
if (StringUtils.isBlank(publicKey) || StringUtils.isBlank(publicKey)) {
Map map = generateKey();
publicKey = map.get("PUB");
privateKey = map.get("PRV");
log.warn("RSAProcessor is not configured with a key pair, use randomly generated key pair.\nPublicKey: {}\nPrivateKey: {}", publicKey, privateKey);
}
this.publicKey = publicKey;
this.privateKey = privateKey;
this.rsa = new RSA(privateKey, publicKey);
}
@Override
public byte[] encrypt(byte[] data) {
return rsa.encrypt(data, KeyType.PublicKey);
}
@Override
public byte[] decrypt(String text) {
return rsa.decrypt(text, KeyType.PrivateKey);
}
/**
* 以base64编码生成秘钥对
*
* @return key="PUB"为公钥 key="PRV"为私钥
*/
public static Map generateKey() {
return generateKey(SecurityMode.BASE64);
}
/**
* 生成RSA秘钥对
* map.get("PUB"):公钥
* map.get("PRV"):私钥
*
* @param type {@link SecurityMode} 编码模式:hex,默认base64
* @return key="PUB"为公钥 key="PRV"为私钥
*/
public static Map generateKey(SecurityMode type) {
Map map = new HashMap<>();
KeyPair pair = SecureUtil.generateKeyPair("RSA");
String PRV;
String PUB;
switch (type) {
case HEX:
PRV = HexUtil.encodeHexStr(pair.getPrivate().getEncoded(), false);
PUB = HexUtil.encodeHexStr(pair.getPublic().getEncoded(), false);
break;
case BASE64:
default:
PRV = Base64Encoder.encode(pair.getPrivate().getEncoded());
PUB = Base64Encoder.encode(pair.getPublic().getEncoded());
}
map.put("PUB", PUB);
map.put("PRV", PRV);
return map;
}
public static void main(String[] args) {
Map map = generateKey();
String PRV = map.get("PRV");
System.out.println("PRV = " + PRV);
String PUB = map.get("PUB");
System.out.println("PUB = " + PUB);
String text = "JAVA";
RSAProcessor converter = new RSAProcessor(null, PRV);
byte[] encrypt = converter.encrypt(StrUtil.bytes(text, CharsetUtil.CHARSET_UTF_8));
String encodeHexStr = HexUtil.encodeHexStr(encrypt, false);
System.out.println(encodeHexStr);
byte[] decrypt = converter.decrypt(encodeHexStr);
System.out.println(StrUtil.str(decrypt, CharsetUtil.CHARSET_UTF_8));
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy