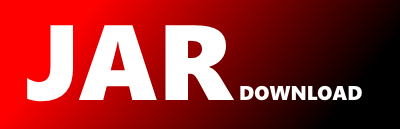
fatjar.implementations.cache.MapCache Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of FatJar Show documentation
Show all versions of FatJar Show documentation
FatJar simple API to quick prototyping and portable web services
Please wait ...
© 2015 - 2025 Weber Informatics LLC | Privacy Policy