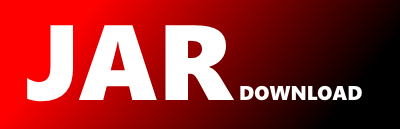
com.fhoster.livebase.AnnotationProcessor Maven / Gradle / Ivy
The newest version!
/**
* Copyright 2024 Fhoster srl
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.fhoster.livebase;
import com.google.common.base.Objects;
import java.lang.annotation.Annotation;
import java.util.HashSet;
import java.util.List;
import java.util.Set;
import javax.annotation.processing.AbstractProcessor;
import javax.annotation.processing.ProcessingEnvironment;
import javax.annotation.processing.RoundEnvironment;
import javax.lang.model.SourceVersion;
import javax.lang.model.element.Element;
import javax.lang.model.element.ElementKind;
import javax.lang.model.element.ExecutableElement;
import javax.lang.model.element.Modifier;
import javax.lang.model.element.PackageElement;
import javax.lang.model.element.TypeElement;
import javax.lang.model.element.VariableElement;
import javax.lang.model.type.DeclaredType;
import javax.lang.model.type.TypeMirror;
import javax.tools.Diagnostic;
import javax.tools.FileObject;
import javax.tools.StandardLocation;
import org.eclipse.xtext.xbase.lib.CollectionLiterals;
import org.eclipse.xtext.xbase.lib.Conversions;
import org.eclipse.xtext.xbase.lib.Exceptions;
import org.eclipse.xtext.xbase.lib.Functions.Function0;
import org.eclipse.xtext.xbase.lib.Functions.Function1;
import org.eclipse.xtext.xbase.lib.IterableExtensions;
import org.eclipse.xtext.xbase.lib.ListExtensions;
import org.eclipse.xtext.xbase.lib.Procedures.Procedure1;
@SuppressWarnings("all")
public abstract class AnnotationProcessor extends AbstractProcessor {
private ProcessingEnvironment processingEnvironment;
private Procedure1 _printer = ((Procedure1) (String message) -> {
this.processingEnvironment.getMessager().printMessage(Diagnostic.Kind.NOTE, message);
});
@Override
public synchronized void init(final ProcessingEnvironment processingEnv) {
this.processingEnvironment = processingEnv;
}
public void setPrinter(final Procedure1 super String> p) {
this._printer = ((Procedure1)p);
}
@Override
public boolean process(final Set extends TypeElement> annotations, final RoundEnvironment env) {
try {
for (final TypeElement te : annotations) {
Set extends Element> _elementsAnnotatedWith = env.getElementsAnnotatedWith(te);
for (final Element e : _elementsAnnotatedWith) {
{
final Annotation annotation = e.getAnnotation(this.supportedAnnotation());
if ((annotation != null)) {
this.processAnnotatedClass(e);
}
}
}
}
} catch (final Throwable _t) {
if (_t instanceof InvalidPluginConfigurationException) {
return true;
} else {
throw Exceptions.sneakyThrow(_t);
}
}
return true;
}
public abstract void processAnnotatedClass(final Element element);
protected String[] extractConstructorTypes(final ExecutableElement constructor) {
final Function1 _function = (VariableElement p) -> {
return p.asType().toString();
};
return ((String[])Conversions.unwrapArray(ListExtensions.map(constructor.getParameters(), _function), String.class));
}
protected CheckElse check(final Element e, final Function0 extends Boolean> condition) {
return new CheckElse(e, condition, this);
}
protected void check(final Element e, final Function0 extends Boolean> condition, final String message) {
try {
Boolean _apply = condition.apply();
boolean _not = (!(_apply).booleanValue());
if (_not) {
this.error(e, message);
throw new InvalidPluginConfigurationException();
}
} catch (Throwable _e) {
throw Exceptions.sneakyThrow(_e);
}
}
protected void error(final Element e, final String msg) {
this.processingEnvironment.getMessager().printMessage(Diagnostic.Kind.ERROR, msg, e);
}
protected void warning(final Element e, final String msg) {
this.processingEnvironment.getMessager().printMessage(Diagnostic.Kind.WARNING, msg, e);
}
@Override
public Set getSupportedAnnotationTypes() {
HashSet _xblockexpression = null;
{
final HashSet annotations = CollectionLiterals.newHashSet();
annotations.add(this.supportedAnnotation().getCanonicalName());
_xblockexpression = annotations;
}
return _xblockexpression;
}
public abstract Class extends Annotation> supportedAnnotation();
@Override
public SourceVersion getSupportedSourceVersion() {
return SourceVersion.latestSupported();
}
protected void info(final String m) {
this._printer.apply(m);
}
protected PackageElement extractPackage(final TypeElement elementInterface) {
return this.processingEnvironment.getElementUtils().getPackageOf(elementInterface);
}
protected FileObject createResource(final StandardLocation location, final String fileName) {
try {
return this.processingEnvironment.getFiler().createResource(location, "", fileName);
} catch (Throwable _e) {
throw Exceptions.sneakyThrow(_e);
}
}
private Iterable constructors(final Element e) {
final Function1 _function = (Element it) -> {
ElementKind _kind = it.getKind();
return Boolean.valueOf(Objects.equal(_kind, ElementKind.CONSTRUCTOR));
};
final Function1 _function_1 = (Element it) -> {
return ((ExecutableElement) it);
};
return IterableExtensions.map(IterableExtensions.filter(((TypeElement) e).getEnclosedElements(), _function), _function_1);
}
private Iterable publicConstructors(final Element e) {
final Function1 _function = (Element it) -> {
return Boolean.valueOf((Objects.equal(it.getKind(), ElementKind.CONSTRUCTOR) && it.getModifiers().contains(Modifier.PUBLIC)));
};
final Function1 _function_1 = (Element it) -> {
return ((ExecutableElement) it);
};
return IterableExtensions.map(IterableExtensions.filter(((TypeElement) e).getEnclosedElements(), _function), _function_1);
}
protected Iterable inectableConstructors(final Element e) {
final Iterable res = this.constructors(e);
int _size = IterableExtensions.size(res);
boolean _lessEqualsThan = (_size <= 1);
if (_lessEqualsThan) {
return res;
}
final Iterable pubRes = this.publicConstructors(e);
int _size_1 = IterableExtensions.size(pubRes);
boolean _equals = (_size_1 == 0);
if (_equals) {
return res;
}
return pubRes;
}
protected ExecutableElement inectableConstructor(final Element e) {
try {
Iterable res = this.inectableConstructors(e);
int _size = IterableExtensions.size(res);
boolean _equals = (_size == 1);
if (_equals) {
return IterableExtensions.head(res);
}
throw new InvalidPluginConfigurationException();
} catch (Throwable _e) {
throw Exceptions.sneakyThrow(_e);
}
}
protected List interfaces(final Element e) {
final Function1 _function = (TypeMirror it) -> {
Element _asElement = ((DeclaredType) it).asElement();
return ((TypeElement) _asElement);
};
return ListExtensions.map(((TypeElement) e).getInterfaces(), _function);
}
protected TypeMirror superclass(final Element e) {
return ((TypeElement) e).getSuperclass();
}
protected boolean isClass(final Element e) {
ElementKind _kind = e.getKind();
return Objects.equal(_kind, ElementKind.CLASS);
}
protected boolean isPublicClass(final Element e) {
return (Objects.equal(e.getKind(), ElementKind.CLASS) && e.getModifiers().contains(Modifier.PUBLIC));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy