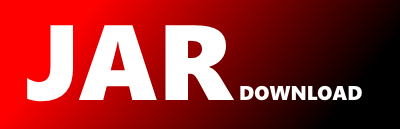
com.fhs.trans.service.impl.DictionaryTransService Maven / Gradle / Ivy
package com.fhs.trans.service.impl;
import com.fhs.cache.service.BothCacheService;
import com.fhs.cache.service.RedisCacheService;
import com.fhs.common.constant.Constant;
import com.fhs.common.utils.JsonUtils;
import com.fhs.common.utils.StringUtil;
import com.fhs.core.trans.anno.Trans;
import com.fhs.core.trans.anno.UnTrans;
import com.fhs.core.trans.constant.TransType;
import com.fhs.core.trans.util.ReflectUtils;
import com.fhs.core.trans.vo.VO;
import com.fhs.trans.fi.LocaleGetter;
import com.fhs.trans.listener.TransMessageListener;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.beans.factory.InitializingBean;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.util.StringUtils;
import java.lang.reflect.Field;
import java.util.*;
import java.util.concurrent.ConcurrentHashMap;
/**
* 字典翻译服务
*
* @author jackwang
* @date 2020-05-18 14:41:20
*/
public class DictionaryTransService implements ITransTypeService, InitializingBean {
private static final String UNTRANS_PREFIX = "un_trans:";
private static final Logger LOGGER = LoggerFactory.getLogger(DictionaryTransService.class);
@Autowired
private BothCacheService bothCacheService;
@Autowired(required = false)
private RedisCacheService redisCacheService;
/**
* 是否开启国际化
*/
private boolean isOpenI18n;
/**
* 当前国际化语言环境获取
*/
private LocaleGetter localeGetter;
/**
* 是否使用redis 默认false
*/
@Value("${easy-trans.dict-use-redis:false}")
private boolean isUseRedis;
/**
* 刷新缓存
*
* @param dictGroupCode 字典分组编码
* @param dicMap 字典map
*/
public void refreshCache(String dictGroupCode, Map dicMap) {
if (isUseRedis && redisCacheService == null) {
throw new RuntimeException("使用redis 请将 easy-trans.is-enable-redis 设置为true");
}
dicMap.keySet().forEach(dictCode -> {
bothCacheService.put(dictGroupCode + "_" + dictCode, dicMap.get(dictCode), false);
bothCacheService.put(UNTRANS_PREFIX + dictGroupCode + "_" + dicMap.get(dictCode), dictCode , false);
});
}
/**
* 刷新缓存并通知其他的微服务清理缓存
*
* @param dictGroupCode 字典分组编码
* @param dicMap 字典map
*/
public void refreshCacheAndNoticeOtherService(String dictGroupCode, Map dicMap) {
//删除完了之后重新插入
bothCacheService.remove(dictGroupCode, false);
bothCacheService.remove(UNTRANS_PREFIX + dictGroupCode, false);
refreshCache(dictGroupCode, dicMap);
noticeOtherService(dictGroupCode);
}
/**
* 删除字典分组缓存并且通知其他的微服务同步删除进程缓存
* @param dictGroupCode 字典分组编码
*/
public void removeDictGroupAndNoticeOtherService(String dictGroupCode){
//删除完了之后重新插入
bothCacheService.remove(dictGroupCode, false);
bothCacheService.remove(UNTRANS_PREFIX + dictGroupCode, false);
noticeOtherService(dictGroupCode);
}
/**
* 刷新单个字典,用于字典项的新增和修改操作
* @param dictGroupCode 分组编码
* @param dictCode 字典编码
* @param dictTransResult 字典翻译结果
*/
public void refreshDictItem(String dictGroupCode,String dictCode,String dictTransResult){
//删除完了之后重新插入
bothCacheService.remove(dictGroupCode + '_' + dictCode, false);
bothCacheService.remove(UNTRANS_PREFIX + '_' + dictTransResult, false);
Map dicMap = new HashMap<>();
dicMap.put(dictCode,dictTransResult);
refreshCache(dictGroupCode, dicMap);
//因为是根据前缀删除,所以这里dictGroupCode+ '_' + dictCode 就只会删除单个了
noticeOtherService(dictGroupCode+ '_' + dictCode);
}
/**
* 通知其他的微服务刷新缓存
* @param dictGroupCode 字典分组编码
*/
public void noticeOtherService(String dictGroupCode) {
Map body = new HashMap<>();
body.put("transType", TransType.DICTIONARY);
body.put("dictGroupCode", dictGroupCode);
redisCacheService.convertAndSend("trans", JsonUtils.map2json(body));
}
/**
* 清理本地缓存
*
* @param messageMap
*/
public void clearCache(Map messageMap) {
String dictGroupCode = StringUtil.toString(messageMap.get("dictGroupCode"));
if (!StringUtils.isEmpty(dictGroupCode)) {
bothCacheService.remove(dictGroupCode, true);
bothCacheService.remove(UNTRANS_PREFIX + dictGroupCode, true);
}
}
public Map getDictionaryTransMap() {
return bothCacheService.getLocalCacheMap();
}
@Override
public void unTransOne(Object obj, List toTransList) {
for (Field tempField : toTransList) {
tempField.setAccessible(true);
UnTrans unTrans = tempField.getAnnotation(UnTrans.class);
try {
String value = bothCacheService.get(UNTRANS_PREFIX + unTrans.dict() + "_"
+ ReflectUtils.getDeclaredField(obj.getClass(),unTrans.refs()[0]).get(obj));
setValue(obj,tempField.getName(),value);
} catch (IllegalAccessException e) {
throw new RuntimeException(e);
}
}
}
@Override
public void unTransMore(List objList, List toTransList) {
for (Object obj : objList) {
unTransOne(obj, toTransList);
}
}
@Override
public void transOne(VO obj, List toTransList) {
Trans tempTrans = null;
for (Field tempField : toTransList) {
tempField.setAccessible(true);
tempTrans = tempField.getAnnotation(Trans.class);
String dicCodes = StringUtil.toString(ReflectUtils.getValue(obj, tempField.getName()));
if(dicCodes.contains("[")){
dicCodes = dicCodes.replace("[", "").replace("]", "");
}
if (dicCodes.contains(",")) {
dicCodes = dicCodes.replace(" ", "");
}
String[] dicCodeArray = dicCodes.split(",");
String key = tempTrans.key().contains("KEY_") ? StringUtil.toString(ReflectUtils.getValue(obj, tempTrans.key().replace("KEY_", ""))) : tempTrans.key();
//sex_0/1 男 女
List dicCodeList = new ArrayList<>(1);
for (String dicCode : dicCodeArray) {
if (!StringUtil.isEmpty(dicCode)) {
dicCodeList.add(bothCacheService.get(getMapKey(key.trim(), dicCode)));
}
}
String transResult = dicCodeList.size() > Constant.ZERO ? StringUtil.getStrForIn(dicCodeList, false) : "";
if (obj.getTransMap() != null && !setRef(tempTrans, obj, transResult)) {
obj.getTransMap().put(tempField.getName() + "Name", transResult);
}
}
}
/**
* 获取map翻译的key
*
* @param dictGroupCode 字典分组编码
* @param dictCode 字典编码
* @return 翻译mapkey
*/
public String getMapKey(String dictGroupCode, String dictCode) {
//开启了国际化就拼接国际化
if (this.isOpenI18n) {
return dictGroupCode + "_" + dictCode + "_" + this.localeGetter.getLanguageTag();
}
return dictGroupCode + "_" + dictCode;
}
@Override
public void transMore(List extends VO> objList, List toTransList) {
for (VO obj : objList) {
transOne(obj, toTransList);
}
}
@Override
public void afterPropertiesSet() throws Exception {
//注册自己为一个服务
TransService.registerTransType(TransType.DICTIONARY, this);
//注册刷新缓存服务
TransMessageListener.regTransRefresher(TransType.DICTIONARY, this::clearCache);
}
/**
* 开启国际化
*
* @param localeGetter
*/
public void openI18n(LocaleGetter localeGetter) {
this.isOpenI18n = true;
this.localeGetter = localeGetter;
}
/**
* 标记使用redis
*/
public void makeUseRedis() {
this.isUseRedis = true;
this.bothCacheService.setUseRedis(true);
if (redisCacheService == null) {
throw new RuntimeException("使用redis 请将 easy-trans.is-enable-redis 设置为true");
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy