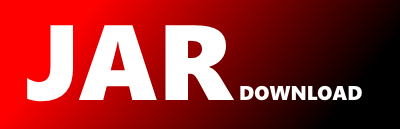
com.fibanez.jsonschema.content.generator.util.ReflectionUtils Maven / Gradle / Ivy
package com.fibanez.jsonschema.content.generator.util;
import com.fibanez.jsonschema.content.Context;
import com.fibanez.jsonschema.content.generator.exception.GeneratorException;
import lombok.AccessLevel;
import lombok.NoArgsConstructor;
import org.reflections.Reflections;
import java.lang.reflect.Constructor;
import java.lang.reflect.Modifier;
import java.lang.reflect.ParameterizedType;
import java.lang.reflect.Type;
import java.util.Set;
import java.util.stream.Stream;
import static java.util.function.Predicate.not;
@NoArgsConstructor(access = AccessLevel.PRIVATE)
public final class ReflectionUtils {
/**
* @return a collection of the classes T
*/
public static Stream> getSubClassesOf(Class clazz) {
String packageName = clazz.getPackage().getName();
Reflections reflections = new Reflections(packageName);
Set> set = reflections.getSubTypesOf(clazz);
return set.stream()
.filter(not(ReflectionUtils::isAbstractClass).and(not(Class::isInterface)));
}
public static boolean isAbstractClass(Class> clazz) {
return Modifier.isAbstract(clazz.getModifiers());
}
public static Type getClassType(Class> clazz) {
try {
Type type;
if (clazz.getGenericInterfaces().length > 0) {
type = clazz.getGenericInterfaces()[0];
} else {
type = clazz.getGenericSuperclass();
}
ParameterizedType parameterizedType = (ParameterizedType) type;
return parameterizedType.getActualTypeArguments()[0];
} catch (Exception e) {
throw new GeneratorException(e, "Could not find out the class type for " + clazz.getCanonicalName());
}
}
/**
* @param clazz generic class
* @param type
* @return A new instance of the class
*/
public static T getDefaultInstanceOf(Class clazz, Context ctx) {
try {
@SuppressWarnings("unchecked")
Constructor[] constructors = (Constructor[]) clazz.getDeclaredConstructors();
for (Constructor c : constructors) {
Class>[] parameterTypes = c.getParameterTypes();
if (parameterTypes.length == 1 && parameterTypes[0] == Context.class) {
c.setAccessible(true);
return c.newInstance(ctx);
}
}
constructors[0].setAccessible(true);
return constructors[0].newInstance();
} catch (Exception e) {
throw new GeneratorException(e, "Could not create a instance of " + clazz.getCanonicalName());
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy