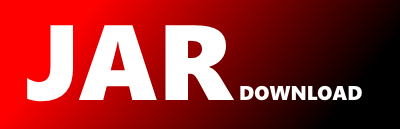
org.fife.rsta.ac.java.PackageMapNode Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of languagesupport Show documentation
Show all versions of languagesupport Show documentation
A library adding code completion and other advanced features for Java, JavaScript, Perl, and other languages to RSyntaxTextArea.
Please wait ...
© 2015 - 2025 Weber Informatics LLC | Privacy Policy