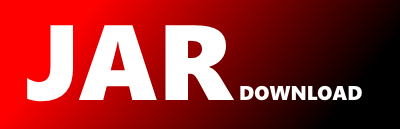
org.fife.rsta.ui.RComboBoxModel Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of rstaui Show documentation
Show all versions of rstaui Show documentation
RSTAUI is an add-on library for RSyntaxTextArea that provides pre-build dialog boxes commonly needed in text editing applications.
/*
* 01/15/2004
*
* RComboBoxModel.java - A combo box model that limits the number of items
* the combo box wil remember. It also won't add an item to the combo box
* if it is already there.
* This library is distributed under a modified BSD license. See the included
* RSTAUI.License.txt file for details.
*/
package org.fife.rsta.ui;
import java.util.Vector;
import javax.swing.DefaultComboBoxModel;
/**
* A combo box model that limits the number of items the combo box will
* "remember." You can use it like so:
*
* RComboBoxModel model = new RComboBoxModel();
* model.setMaxNumElements(10);
* JComboBox comboBox = new JComboBox(model);
* It also won't let you add an item to the combo box twice (i.e., no
* duplicates), and it adds new items to the beginning of the list, not
* the end (as JComboBox
's do by default).
* It defaults to 8 elements remembered.
*
* @author Robert Futrell
* @version 0.8
*/
public class RComboBoxModel extends DefaultComboBoxModel {
private static final long serialVersionUID = 1L;
/**
* The number of items the combo box will remember.
*/
private int maxNumElements;
/**
* Creates a new combo box model with a maximum element count of
* 8
.
*/
public RComboBoxModel() {
setMaxNumElements(8);
}
/**
* Creates a new combo box model with a maximum element count of
* 8
.
*
* @param items The initial items to use to populate the combo box.
*/
public RComboBoxModel(Object[] items) {
super(items);
setMaxNumElements(8);
}
/**
* Creates a new combo box model with a maximum element count of
* 8
.
*
* @param v The initial items to use to populate the combo box.
*/
public RComboBoxModel(Vector> v) {
super(v);
setMaxNumElements(8);
}
/**
* Adds the object (if it's not already in the list) to the front of
* the list. If it's already in the list, move it to the top.
*
* @param anObject The object to add.
*/
@Override
public void addElement(Object anObject) {
insertElementAt(anObject, 0);
}
/**
* Ensures the number if items remembered by this combo box is valid.
*/
private void ensureValidItemCount() {
while (getSize()>maxNumElements)
removeElementAt(getSize()-1);
}
/**
* Returns the maximum number of items this combo box can hold.
*
* @return The maximum number of items this combo box can hold.
*/
public int getMaxNumElements() {
return maxNumElements;
}
/**
* Adds an item at a specified index. The implementation of this method
* should notify all registered ListDataListeners
that the
* item has been added.
*
* @param anObject The Object
to be added.
* @param index Location to add the object.
*/
@Override
public void insertElementAt(Object anObject, int index) {
int oldPos = getIndexOf(anObject);
if (oldPos==index) { // Already at the desired location.
return;
}
if (oldPos>-1) { // Remove it first if it's somewhere else.
removeElement(anObject);
}
super.insertElementAt(anObject, index);
ensureValidItemCount();
}
/**
* Sets the maximum number of items this combo box can hold.
*
* @param numElements The maximum number of items this combo box can hold.
* If numElements <= 0
, then the capacity
* of this combo box is set to 4
.
*/
public void setMaxNumElements(int numElements) {
maxNumElements = numElements<=0 ? 4 : numElements;
ensureValidItemCount();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy