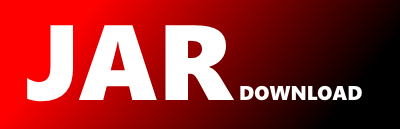
org.fife.ui.rsyntaxtextarea.StyledTextTransferable Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of rsyntaxtextarea Show documentation
Show all versions of rsyntaxtextarea Show documentation
RSyntaxTextArea is the syntax highlighting text editor for Swing applications. Features include syntax highlighting for 40+ languages, code folding, code completion, regex find and replace, macros, code templates, undo/redo, line numbering and bracket matching.
The newest version!
/*
* 07/28/2008
*
* StyledTextTransferable.java - Used during drag-and-drop to represent RTF text.
*
* This library is distributed under a modified BSD license. See the included
* LICENSE file for details.
*/
package org.fife.ui.rsyntaxtextarea;
import java.awt.datatransfer.DataFlavor;
import java.awt.datatransfer.Transferable;
import java.awt.datatransfer.UnsupportedFlavorException;
import java.io.ByteArrayInputStream;
import java.io.IOException;
import java.io.StringReader;
/**
* Object used during copy/paste and DnD operations to represent styled text.
* It can return the text being moved as HTML, RTF or plain text. This
* class is basically the same as
* java.awt.datatransfer.StringSelection
, except that it can also
* return the text in a couple of styled text formats.
*
* @author Robert Futrell
* @version 1.0
*/
class StyledTextTransferable implements Transferable {
/**
* The transferred plain text.
*/
private String plain;
/**
* The transferred text, as HTML.
*/
private String html;
/**
* The RTF data, in bytes (the RTF is 7-bit ascii).
*/
private byte[] rtfBytes;
/**
* The "flavors" the text can be returned as.
*/
private static final DataFlavor[] FLAVORS = {
DataFlavor.fragmentHtmlFlavor,
new DataFlavor("text/rtf", "RTF"),
DataFlavor.stringFlavor,
DataFlavor.plainTextFlavor // deprecated
};
/**
* Constructor.
*
* @param html The transferred text, as HTML.
* @param rtfBytes The transferred text, as RTF bytes.
*/
StyledTextTransferable(String plain, String html, byte[] rtfBytes) {
this.plain = plain;
this.html = html;
this.rtfBytes = rtfBytes;
}
@Override
public Object getTransferData(DataFlavor flavor)
throws UnsupportedFlavorException, IOException {
if (flavor.equals(FLAVORS[0])) { // HTML
return html;
}
else if (flavor.equals(FLAVORS[1])) { // RTF
return new ByteArrayInputStream(rtfBytes ==null ? new byte[0] : rtfBytes);
}
else if (flavor.equals(FLAVORS[2])) { // stringFlavor
return plain;
}
else if (flavor.equals(FLAVORS[3])) { // plainTextFlavor (deprecated)
return new StringReader(plain);
}
throw new UnsupportedFlavorException(flavor);
}
@Override
public DataFlavor[] getTransferDataFlavors() {
return FLAVORS.clone();
}
@Override
public boolean isDataFlavorSupported(DataFlavor flavor) {
for (DataFlavor flavor1 : FLAVORS) {
if (flavor.equals(flavor1)) {
return true;
}
}
return false;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy