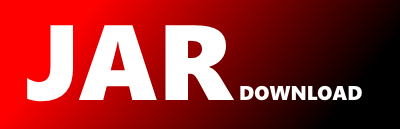
org.fife.ui.rsyntaxtextarea.TokenMakerFactory Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of rsyntaxtextarea Show documentation
Show all versions of rsyntaxtextarea Show documentation
RSyntaxTextArea is the syntax highlighting text editor for Swing applications. Features include syntax highlighting for 40+ languages, code folding, code completion, regex find and replace, macros, code templates, undo/redo, line numbering and bracket matching.
The newest version!
/*
* 12/12/2008
*
* TokenMakerFactory.java - A factory for TokenMakers.
*
* This library is distributed under a modified BSD license. See the included
* LICENSE file for details.
*/
package org.fife.ui.rsyntaxtextarea;
import java.util.Set;
import org.fife.ui.rsyntaxtextarea.modes.PlainTextTokenMaker;
/**
* A factory that maps syntax styles to {@link TokenMaker}s capable of splitting
* text into tokens for those syntax styles.
*
* @author Robert Futrell
* @version 1.0
*/
public abstract class TokenMakerFactory {
/**
* If this system property is set, a custom TokenMakerFactory
* of the specified class will be used as the default token maker factory.
*/
public static final String PROPERTY_DEFAULT_TOKEN_MAKER_FACTORY =
"TokenMakerFactory";
/**
* The singleton default TokenMakerFactory
instance.
*/
private static TokenMakerFactory DEFAULT_INSTANCE;
/**
* Returns the default TokenMakerFactory
instance. This is
* the factory used by all {@link RSyntaxDocument}s by default.
*
* @return The factory.
* @see #setDefaultInstance(TokenMakerFactory)
*/
public static synchronized TokenMakerFactory getDefaultInstance() {
if (DEFAULT_INSTANCE==null) {
String clazz;
try {
clazz= System.getProperty(PROPERTY_DEFAULT_TOKEN_MAKER_FACTORY);
} catch (java.security.AccessControlException ace) {
clazz = null; // We're in an applet; take default.
}
if (clazz==null) {
clazz = "org.fife.ui.rsyntaxtextarea.DefaultTokenMakerFactory";
}
try {
DEFAULT_INSTANCE = (TokenMakerFactory)Class.forName(clazz).
getDeclaredConstructor().newInstance();
} catch (RuntimeException re) { // FindBugs
throw re;
} catch (Exception e) {
e.printStackTrace();
throw new InternalError("Cannot find TokenMakerFactory: " +
clazz);
}
}
return DEFAULT_INSTANCE;
}
/**
* Returns a {@link TokenMaker} for the specified key.
*
* @param key The key.
* @return The corresponding TokenMaker
, or
* {@link PlainTextTokenMaker} if none matches the specified key.
*/
public final TokenMaker getTokenMaker(String key) {
TokenMaker tm = getTokenMakerImpl(key);
if (tm==null) {
tm = new PlainTextTokenMaker();
}
return tm;
}
/**
* Returns a {@link TokenMaker} for the specified key.
*
* @param key The key.
* @return The corresponding TokenMaker
, or null
* if none matches the specified key.
*/
protected abstract TokenMaker getTokenMakerImpl(String key);
/**
* Returns the set of keys that this factory maps to token makers.
*
* @return The set of keys.
*/
public abstract Set keySet();
/**
* Sets the default TokenMakerFactory
instance. This is
* the factory used by all future {@link RSyntaxDocument}s by default.
* RSyntaxDocument
s that have already been created are not
* affected.
*
* @param tmf The factory.
* @throws IllegalArgumentException If tmf
is
* null
.
* @see #getDefaultInstance()
*/
public static synchronized void setDefaultInstance(TokenMakerFactory tmf) {
if (tmf==null) {
throw new IllegalArgumentException("tmf cannot be null");
}
DEFAULT_INSTANCE = tmf;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy