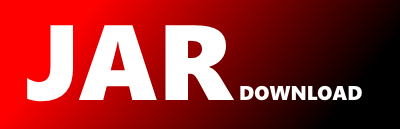
org.fife.util.DynamicIntArray Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of rsyntaxtextarea Show documentation
Show all versions of rsyntaxtextarea Show documentation
RSyntaxTextArea is the syntax highlighting text editor for Swing applications. Features include syntax highlighting for 40+ languages, code folding, code completion, regex find and replace, macros, code templates, undo/redo, line numbering and bracket matching.
The newest version!
/* * 03/26/2004 * * DynamicIntArray.java - Similar to an ArrayList, but holds ints instead * of Objects. * * This library is distributed under a modified BSD license. See the included * LICENSE file for details. */ package org.fife.util; import java.io.Serializable; import java.util.Arrays; /** * Similar to a
instance can hold * at least the number of elements specified. If it can't, then the * capacity is increased. * * @param minCapacity The desired minimum capacity. */ private void ensureCapacity(int minCapacity) { int oldCapacity = data.length; if (minCapacity > oldCapacity) { int[] oldData = data; // Ensures we don't just keep increasing capacity by some small // number like 1... int newCapacity = (oldCapacity * 3)/2 + 1; if (newCapacity < minCapacity) { newCapacity = minCapacity; } data = new int[newCapacity]; System.arraycopy(oldData,0, data,0, size); } } /** * Sets the value of all entries in this array to the specified value. * * @param value The new value for all elements in the array. */ public void fill(int value) { Arrays.fill(data, value); } /** * Returns thejava.util.ArrayList
, but specifically for *int
s. This is basically an array of integers that resizes * itself (if necessary) when adding new elements. * * @author Robert Futrell * @version 0.8 */ public class DynamicIntArray implements Serializable { /** * The actual data. */ private int[] data; /** * The number of values in the array. Note that this is NOT the * capacity of the array; rather,size <= capacity
. */ private int size; /** * Constructs a new array object with an initial capacity of 10. */ public DynamicIntArray() { this(10); } /** * Constructs a new array object with a given initial capacity. * * @param initialCapacity The initial capacity. * @throws IllegalArgumentException IfinitialCapacity
is * negative. */ public DynamicIntArray(int initialCapacity) { if (initialCapacity<0) { throw new IllegalArgumentException("Illegal initialCapacity: " + initialCapacity); } data = new int[initialCapacity]; size = 0; } /** * Constructs a new array object from the given int array. The resulting *DynamicIntArray
will have an initial capacity of 110% * the size of the array. * * @param intArray Initial data for the array object. * @throws NullPointerException IfintArray
is *null
. */ public DynamicIntArray(int[] intArray) { size = intArray.length; int capacity = (int)Math.min(size*110L/100, Integer.MAX_VALUE); data = new int[capacity]; System.arraycopy(intArray,0, data,0, size); // source, dest, length. } /** * Appends the specifiedint
to the end of this array. * * @param value Theint
to be appended to this array. */ public void add(int value) { ensureCapacity(size + 1); data[size++] = value; } /** * Inserts allint
s in the specified array into this array * object at the specified location. Shifts theint
* currently at that position (if any) and any subsequent *int
s to the right (adds one to their indices). * * @param index The index at which the specified integer is to be * inserted. * @param intArray The array ofint
s to insert. * @throws IndexOutOfBoundsException Ifindex
is less than * zero or greater thangetSize()
. * @throws NullPointerException IfintArray
is *null
. */ public void add(int index, int[] intArray) { if (index>size) { throwException2(index); } int addCount = intArray.length; ensureCapacity(size+addCount); int moveCount = size - index; if (moveCount>0) { System.arraycopy(data,index, data,index+addCount, moveCount); } System.arraycopy(intArray,0, data,index, addCount); size += addCount; } /** * Inserts the specifiedint
at the specified position in * this array. Shifts theint
currently at that position (if * any) and any subsequentint
s to the right (adds one to * their indices). * * @param index The index at which the specified integer is to be * inserted. * @param value Theint
to be inserted. * @throws IndexOutOfBoundsException Ifindex
is less than * zero or greater thangetSize()
. */ public void add(int index, int value) { if (index>size) { throwException2(index); } ensureCapacity(size+1); System.arraycopy(data,index, data,index+1, size-index); data[index] = value; size++; } /** * Removes all values from this array object. Capacity will remain the * same. */ public void clear() { size = 0; } /** * Returns whether this array contains a given integer. This method * performs a linear search, so it is not optimized for performance. * * @param integer Theint
for which to search. * @return Whether the given integer is contained in this array. */ public boolean contains(int integer) { for (int i=0; iDynamicIntArray int
at the specified position in this array * object. * * @param index The index of theint
to return. * @return Theint
at the specified position in this array. * @throws IndexOutOfBoundsException Ifindex
is less than * zero or greater than or equal togetSize()
. */ public int get(int index) { // Small enough to be inlined, and throwException() is rarely called. if (index>=size) { throwException(index); } return data[index]; } /** * Returns theint
at the specified position in this array * object, without doing any bounds checking. You really should use * {@link #get(int)} instead of this method. * * @param index The index of theint
to return. * @return Theint
at the specified position in this array. */ public int getUnsafe(int index) { // Small enough to be inlined. return data[index]; } /** * Returns the number ofint
s in this array object. * * @return The number ofint
s in this array object. */ public int getSize() { return size; } /** * Increments all values in the array in the specified range. * * @param from The range start offset (inclusive). * @param to The range end offset (exclusive). * @see #decrement(int, int) */ public void increment(int from, int to) { for (int i=from; isize) { throwException2(offs); } ensureCapacity(size+count); System.arraycopy(data,offs, data,offs+count, size-offs); Arrays.fill(data, offs, offs+count, value); size += count; } /** * Returns whether this array object is empty. * * @return Whether this array object contains no elements. */ public boolean isEmpty() { return size==0; } /** * Removes the int
at the specified location from this array * object. * * @param index The index of theint
to remove. * @throws IndexOutOfBoundsException Ifindex
is less than * zero or greater than or equal togetSize()
. */ public void remove(int index) { if (index>=size) { throwException(index); } int toMove = size - index - 1; if (toMove>0) { System.arraycopy(data,index+1, data,index, toMove); } --size; } /** * Removes theint
s in the specified range from this array * object. * * @param fromIndex The index of the firstint
to remove. * @param toIndex The index AFTER the lastint
to remove. * @throws IndexOutOfBoundsException If either offromIndex
* ortoIndex
is less than zero or greater than or * equal togetSize()
. */ public void removeRange(int fromIndex, int toIndex) { if (fromIndex>=size || toIndex>size) { throwException3(fromIndex, toIndex); } int moveCount = size - toIndex; System.arraycopy(data,toIndex, data,fromIndex, moveCount); size -= (toIndex - fromIndex); } /** * Sets theint
value at the specified position in this * array object. * * @param index The index of theint
to set * @param value The value to set it to. * @throws IndexOutOfBoundsException Ifindex
is less than * zero or greater than or equal togetSize()
. */ public void set(int index, int value) { // Small enough to be inlined, and throwException() is rarely called. if (index>=size) { throwException(index); } data[index] = value; } /** * Sets theint
value at the specified position in this * array object, without doing any bounds checking. You should use * {@link #set(int, int)} instead of this method. * * @param index The index of theint
to set * @param value The value to set it to. */ public void setUnsafe(int index, int value) { // Small enough to be inlined. data[index] = value; } /** * Throws an exception. This method isolates error-handling code from * the error-checking code, so that callers (e.g. {@link #get} and * {@link #set}) can be both small enough to be inlined, as well as * not usually make any expensive method calls (since their callers will * usually not pass illegal arguments to them). * * See * this Sun bug report for more information. * * @param index The invalid index. * @throws IndexOutOfBoundsException Always. */ private void throwException(int index) { throw new IndexOutOfBoundsException("Index " + index + " not in valid range [0-" + (size-1) + "]"); } /** * Throws an exception. This method isolates error-handling code from * the error-checking code, so that callers can be both small enough to be * inlined, as well as not usually make any expensive method calls (since * their callers will usually not pass illegal arguments to them). * * See * this Sun bug report for more information. * * @param index The invalid index. * @throws IndexOutOfBoundsException Always. */ private void throwException2(int index) { throw new IndexOutOfBoundsException("Index " + index + ", not in range [0-" + size + "]"); } /** * Throws an exception. This method isolates error-handling code from * the error-checking code, so that callers can be both small enough to be * inlined, as well as not usually make any expensive method calls (since * their callers will usually not pass illegal arguments to them). * * See * this Sun bug report for more information. * * @param fromIndex The from-index. * @param toIndex The to-index. * @throws IndexOutOfBoundsException Always. */ private void throwException3(int fromIndex, int toIndex) { throw new IndexOutOfBoundsException("Index range [" + fromIndex + ", " + toIndex + "] not in valid range [0-" + (size-1) + "]"); } }
© 2015 - 2025 Weber Informatics LLC | Privacy Policy