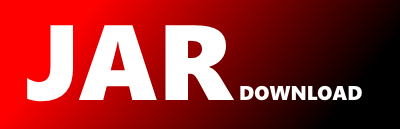
com.files.models.Folder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of files-sdk Show documentation
Show all versions of files-sdk Show documentation
The Files.com Java client library provides convenient access to the Files.com API from JVM based applications.
package com.files.models;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.core.JsonProcessingException;
import com.fasterxml.jackson.core.type.TypeReference;
import com.fasterxml.jackson.databind.json.JsonMapper;
import com.fasterxml.jackson.databind.MapperFeature;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.fasterxml.jackson.databind.ObjectReader;
import com.files.FilesClient;
import com.files.FilesConfig;
import com.files.net.HttpMethods.RequestMethods;
import com.files.util.ModelUtils;
import com.files.util.FilesInputStream;
import java.io.BufferedInputStream;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.FileInputStream;
import java.io.InputStream;
import java.io.OutputStream;
import java.net.URI;
import java.net.URISyntaxException;
import java.util.Date;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import lombok.Getter;
import lombok.Setter;
public class Folder {
private HashMap options;
private ObjectMapper objectMapper = JsonMapper
.builder()
.disable(MapperFeature.CAN_OVERRIDE_ACCESS_MODIFIERS)
.build();
public Folder() {
this(null, null);
}
public Folder(HashMap parameters) {
this(parameters, null);
}
public Folder(HashMap parameters, HashMap options) {
this.options = options;
try{
ObjectReader objectReader = objectMapper.readerForUpdating(this);
objectReader.readValue(objectMapper.writeValueAsString(parameters));
} catch (JsonProcessingException e){
// TODO: error generation on constructor
}
}
/**
* File/Folder path This must be slash-delimited, but it must neither start nor end with a slash. Maximum of 5000 characters.
*/
@Getter
@Setter
@JsonProperty("path")
public String path;
/**
* File/Folder display name
*/
@Getter
@Setter
@JsonProperty("display_name")
public String displayName;
/**
* Type: `directory` or `file`.
*/
@Getter
@Setter
@JsonProperty("type")
public String type;
/**
* File/Folder size
*/
@Getter
@Setter
@JsonProperty("size")
public Long size;
/**
* File created date/time
*/
@Getter
@JsonProperty("created_at")
public Date createdAt;
/**
* File last modified date/time, according to the server. This is the timestamp of the last Files.com operation of the file, regardless of what modified timestamp was sent.
*/
@Getter
@Setter
@JsonProperty("mtime")
public Date mtime;
/**
* File last modified date/time, according to the client who set it. Files.com allows desktop, FTP, SFTP, and WebDAV clients to set modified at times. This allows Desktop :Cloud syncing to preserve modified at times.
*/
@Getter
@Setter
@JsonProperty("provided_mtime")
public Date providedMtime;
/**
* File CRC32 checksum. This is sometimes delayed, so if you get a blank response, wait and try again.
*/
@Getter
@Setter
@JsonProperty("crc32")
public String crc32;
/**
* File MD5 checksum. This is sometimes delayed, so if you get a blank response, wait and try again.
*/
@Getter
@Setter
@JsonProperty("md5")
public String md5;
/**
* MIME Type. This is determined by the filename extension and is not stored separately internally.
*/
@Getter
@Setter
@JsonProperty("mime_type")
public String mimeType;
/**
* Region location
*/
@Getter
@Setter
@JsonProperty("region")
public String region;
/**
* A short string representing the current user's permissions. Can be `r`,`w`,`d`, `l` or any combination
*/
@Getter
@Setter
@JsonProperty("permissions")
public String permissions;
/**
* Are subfolders locked and unable to be modified?
*/
@Getter
@Setter
@JsonProperty("subfolders_locked?")
public Boolean subfoldersLocked;
/**
* Is this folder locked and unable to be modified?
*/
@Getter
@Setter
@JsonProperty("is_locked")
public Boolean isLocked;
/**
* Link to download file. Provided only in response to a download request.
*/
@Getter
@Setter
@JsonProperty("download_uri")
public String downloadUri;
/**
* Bookmark/priority color of file/folder
*/
@Getter
@Setter
@JsonProperty("priority_color")
public String priorityColor;
/**
* File preview ID
*/
@Getter
@Setter
@JsonProperty("preview_id")
public Long previewId;
/**
* File preview
*/
@Getter
@Setter
@JsonProperty("preview")
public Preview preview;
/**
* Create parent directories if they do not exist?
*/
@Getter
@Setter
@JsonProperty("mkdir_parents")
public Boolean mkdirParents;
public void save() throws IOException {
HashMap parameters = ModelUtils.toParameterMap(objectMapper.writeValueAsString(this));
if (parameters.containsKey("id") && parameters.get("id") != null) {
throw new UnsupportedOperationException("The Folder Object doesn't support updates.");
} else {
Folder newObject = Folder.create(parameters, this.options);
}
}
/**
* Parameters:
* cursor - string - Send cursor to resume an existing list from the point at which you left off. Get a cursor from an existing list via the X-Files-Cursor-Next header or the X-Files-Cursor-Prev header.
* per_page - int64 - Number of records to show per page. (Max: 10,000, 1,000 or less is recommended).
* path (required) - string - Path to operate on.
* filter - string - If specified, will filter folders/files list by this string. Wildcards of `*` and `?` are acceptable here.
* preview_size - string - Request a preview size. Can be `small` (default), `large`, `xlarge`, or `pdf`.
* search - string - If `search_all` is `true`, provide the search string here. Otherwise, this parameter acts like an alias of `filter`.
* search_all - boolean - Search entire site? If set, we will ignore the folder path provided and search the entire site. This is the same API used by the search bar in the UI. Search results are a best effort, not real time, and not guaranteed to match every file. This field should only be used for ad-hoc (human) searching, and not as part of an automated process.
* with_previews - boolean - Include file previews?
* with_priority_color - boolean - Include file priority color information?
*/
public static List listFor() throws IOException {
return listFor(null, null,null);
}
public static List listFor(String path, HashMap parameters) throws IOException {
return listFor(path, parameters, null);
}
public static List listFor(HashMap parameters, HashMap options) throws IOException {
return listFor(null, parameters, options);
}
public static List listFor(String path, HashMap parameters, HashMap options) throws IOException {
parameters = parameters != null ? parameters : new HashMap();
options = options != null ? options : new HashMap();
if (path == null && parameters.containsKey("path") && parameters.get("path") != null) {
path = ((String) parameters.get("path"));
}
if (parameters.containsKey("cursor") && !(parameters.get("cursor") instanceof String )) {
throw new IllegalArgumentException("Bad parameter: cursor must be of type String parameters[\"cursor\"]");
}
if (parameters.containsKey("per_page") && !(parameters.get("per_page") instanceof Long )) {
throw new IllegalArgumentException("Bad parameter: per_page must be of type Long parameters[\"per_page\"]");
}
if (!(path instanceof String) ) {
throw new IllegalArgumentException("Bad parameter: path must be of type String parameters[\"path\"]");
}
if (parameters.containsKey("filter") && !(parameters.get("filter") instanceof String )) {
throw new IllegalArgumentException("Bad parameter: filter must be of type String parameters[\"filter\"]");
}
if (parameters.containsKey("preview_size") && !(parameters.get("preview_size") instanceof String )) {
throw new IllegalArgumentException("Bad parameter: preview_size must be of type String parameters[\"preview_size\"]");
}
if (parameters.containsKey("search") && !(parameters.get("search") instanceof String )) {
throw new IllegalArgumentException("Bad parameter: search must be of type String parameters[\"search\"]");
}
if (parameters.containsKey("search_all") && !(parameters.get("search_all") instanceof Boolean )) {
throw new IllegalArgumentException("Bad parameter: search_all must be of type Boolean parameters[\"search_all\"]");
}
if (parameters.containsKey("with_previews") && !(parameters.get("with_previews") instanceof Boolean )) {
throw new IllegalArgumentException("Bad parameter: with_previews must be of type Boolean parameters[\"with_previews\"]");
}
if (parameters.containsKey("with_priority_color") && !(parameters.get("with_priority_color") instanceof Boolean )) {
throw new IllegalArgumentException("Bad parameter: with_priority_color must be of type Boolean parameters[\"with_priority_color\"]");
}
if (path == null) {
throw new NullPointerException("Argument or Parameter missing: path parameters[\"path\"]");
}
String urlParts[] = {FilesConfig.getInstance().getApiRoot(), FilesConfig.getInstance().getApiBase(), path};
for (int i = 2; i < urlParts.length; i++) {
try {
urlParts[i] = new URI(null, null, urlParts[i], null).getRawPath();
} catch (URISyntaxException ex){
}
}
String url = String.format("%s%s/folders/%s", urlParts);
TypeReference> typeReference = new TypeReference>() {};
return FilesClient.requestList(url, RequestMethods.GET, typeReference, parameters, options);
}
/**
* Parameters:
* path (required) - string - Path to operate on.
* mkdir_parents - boolean - Create parent directories if they do not exist?
*/
public static Folder create() throws IOException {
return create(null, null,null);
}
public static Folder create(String path, HashMap parameters) throws IOException {
return create(path, parameters, null);
}
public static Folder create(HashMap parameters, HashMap options) throws IOException {
return create(null, parameters, options);
}
public static Folder create(String path, HashMap parameters, HashMap options) throws IOException {
parameters = parameters != null ? parameters : new HashMap();
options = options != null ? options : new HashMap();
if (path == null && parameters.containsKey("path") && parameters.get("path") != null) {
path = ((String) parameters.get("path"));
}
if (!(path instanceof String) ) {
throw new IllegalArgumentException("Bad parameter: path must be of type String parameters[\"path\"]");
}
if (parameters.containsKey("mkdir_parents") && !(parameters.get("mkdir_parents") instanceof Boolean )) {
throw new IllegalArgumentException("Bad parameter: mkdir_parents must be of type Boolean parameters[\"mkdir_parents\"]");
}
if (path == null) {
throw new NullPointerException("Argument or Parameter missing: path parameters[\"path\"]");
}
String urlParts[] = {FilesConfig.getInstance().getApiRoot(), FilesConfig.getInstance().getApiBase(), path};
for (int i = 2; i < urlParts.length; i++) {
try {
urlParts[i] = new URI(null, null, urlParts[i], null).getRawPath();
} catch (URISyntaxException ex){
}
}
String url = String.format("%s%s/folders/%s", urlParts);
TypeReference typeReference = new TypeReference() {};
return FilesClient.requestItem(url, RequestMethods.POST, typeReference, parameters, options);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy